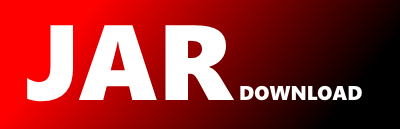
commonMain.com.careem.mockingbird.test.Capture.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mockingbird-jvm Show documentation
Show all versions of mockingbird-jvm Show documentation
A Koltin multiplatform library that provides an easier way to mock and write unit tests for a multiplatform project
/**
*
* Copyright Careem, an Uber Technologies Inc. company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.careem.mockingbird.test
import co.touchlab.stately.collections.ConcurrentMutableList
import kotlinx.atomicfu.AtomicRef
import kotlinx.atomicfu.atomic
/**
* A interface for all capture-able class to implement so the captured value can be stored
*/
public interface Captureable {
public fun storeCapturedValue(value: Any?)
}
/**
* Capture any [Slot] which will be using to compare the property inside
*/
public fun capture(slot: Slot): CapturedMatcher {
return CapturedMatcher(slot)
}
/**
* Capture any [Slot] which will be using to compare the property inside
*/
public fun capture(list: CapturedList): CapturedMatcher {
return CapturedMatcher(list)
}
public interface Slot : Captureable {
public val captured: T?
}
private class ThreadSafeSlot : Slot {
private val _captured: AtomicRef = atomic(null)
override val captured: T?
get() = _captured.value
@Suppress("UNCHECKED_CAST")
override fun storeCapturedValue(value: Any?) {
_captured.value = value as T
}
}
private class LocalThreadSlot : Slot {
private var _captured: T? = null
override var captured: T?
get() {
return _captured
}
private set(value) {
_captured = value
}
@Suppress("UNCHECKED_CAST")
override fun storeCapturedValue(value: Any?) {
this.captured = value as T
}
}
@Deprecated(
message = "Use different function call instead",
replaceWith = ReplaceWith("slot()", "com.careem.mockingbird.test.slot"),
level = DeprecationLevel.WARNING
)
/**
* A slot using to fetch the method invocation and compare the property inside invocation arguments
* Usage example @see [FunctionsTest]
*/
public fun Slot(): Slot {
return slot()
}
/**
* A slot using to fetch the method invocation and compare the property inside invocation arguments
* Usage example @see [FunctionsTest]
*/
public fun slot(): Slot = ThreadSafeSlot()
public interface CapturedList : Captureable {
public val captured: List
}
@Deprecated(
message = "Use different function call instead",
replaceWith = ReplaceWith("capturedList()", "com.careem.mockingbird.test.capturedList"),
level = DeprecationLevel.WARNING
)
/**
* A list that using to fetch the method invocation and compare the property inside
* invocation arguments
* Usage example @see [FunctionsTest]
*/
public fun CapturedList(): CapturedList {
return capturedList()
}
/**
* A list that using to fetch the method invocation and compare the property inside
* invocation arguments
* Usage example @see [FunctionsTest]
*/
public fun capturedList(): CapturedList {
return ThreadSafeCapturedList()
}
private class ThreadSafeCapturedList : CapturedList {
private val _captured = ConcurrentMutableList()
override val captured: List
get() {
return _captured.toList()
}
@Suppress("UNCHECKED_CAST")
override fun storeCapturedValue(value: Any?) {
_captured.add(value as T)
}
}
/**
* A placeholder for where using any() as a testing matcher
*/
public class AnyMatcher
/**
* A placeholder to indicate this argument is captured by [Slot] or [CapturedList]
* Usage example @see [FunctionsTest]
*/
public class CapturedMatcher {
private val captureable: Captureable
public constructor(slot: Slot) {
captureable = slot
}
public constructor(list: CapturedList) {
captureable = list
}
internal fun setCapturedValue(value: Any?) {
captureable.storeCapturedValue(value)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy