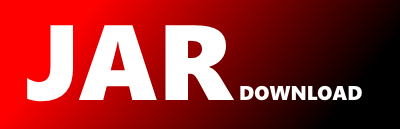
com.carrotgarden.maven.scalor.scalanative.Params.scala Maven / Gradle / Ivy
package com.carrotgarden.maven.scalor.scalanative
import java.io.File
import org.apache.maven.plugins.annotations.Parameter
import org.apache.maven.plugins.annotations.Component
import com.carrotgarden.maven.scalor.base
import com.carrotgarden.maven.tools.Description
import com.carrotgarden.maven.scalor.util.Folder
import com.carrotgarden.maven.scalor.util.Error.Throw
import org.apache.maven.archiver.MavenArchiveConfiguration
import org.apache.maven.project.MavenProjectHelper
import org.apache.maven.project.MavenProject
trait ParamsLinkAny extends AnyRef
with Build
with ParamsRegex
with ParamsLibrary
with ParamsLogging
with ParamsOptions
with ParamsEntryClassMain
with ParamsGarbageCollectorAny {
def nativeWorkdir : File
}
trait ParamsOptions extends AnyRef
with base.ParamsAny {
@Description( """
Linking behaviour for placeholder methods annotated with @stub
.
By default stubs are not linked and are shown as linking errors.
""" )
@Parameter(
property = "scalor.nativeOptionLinkStubs",
defaultValue = "false"
)
var nativeOptionLinkStubs : Boolean = _
@Description( """
LLVM compile options for mode=release.
Uses optimization by default.
These options are added to auto-discovered LLVM options.
Separator parameter: commonSequenceSeparator.
""" )
@Parameter(
property = "scalor.nativeOptionsCompileRelease",
defaultValue = "-O2 ★"
)
var nativeOptionsCompileRelease : String = _
@Description( """
LLVM compile options for mode=debug.
Uses no optimization by default.
These options are added to auto-discovered LLVM options.
Separator parameter: commonSequenceSeparator.
""" )
@Parameter(
property = "scalor.nativeOptionsCompileDebug",
defaultValue = "-O0 ★"
)
var nativeOptionsCompileDebug : String = _
@Description( """
LLVM linking options for mode=release.
Strips symbols by default.
These options are added to auto-discovered LLVM options.
Separator parameter: commonSequenceSeparator.
""" )
@Parameter(
property = "scalor.nativeOptionsLinkingRelease",
defaultValue = "-lpthread ★ -Wl,-s ★"
)
var nativeOptionsLinkingRelease : String = _
@Description( """
LLVM linking options for mode=release.
Keeps symbols by default.
These options are added to auto-discovered LLVM options.
Separator parameter: commonSequenceSeparator.
""" )
@Parameter(
property = "scalor.nativeOptionsLinkingDebug",
defaultValue = "-lpthread ★"
)
var nativeOptionsLinkingDebug : String = _
@Description( """
Options used by objcopy
tool during C data resource embedding.
These options are added to required hard-coded objcopy
invocation options.
Empty by default.
Separator parameter: commonSequenceSeparator.
""" )
@Parameter(
property = "scalor.nativeOptionsObjcopy",
defaultValue = " ★"
)
var nativeOptionsObjcopy : String = _
@Description( """
Mapping required for objcopy
embedder.
Used to convert from LLVM triplet
into GNU objcopy binary-architecture/output
.
LLVM triplet reference.
GNU objcopy --info reference.
Separator parameter: commonSequenceSeparator.
Mapping parameter: commonMappingPattern.
Mapping format, where
triplet-regex
is regular expressoin mathced against LLVM triplet,
binary-architecture/output
is slash-separated pair describing GNU objcopy type:
triplet-regex = binary-architecture/output
""" )
@Parameter(
property = "scalor.nativeObjcopyMapping",
defaultValue = """
x86-([^-]+)-linux-([^-]+) = i386/elf32-i386 ★
x86_64-([^-]+)-linux-([^-]+) = i386/elf64-x86-64 ★
"""
)
var nativeObjcopyMapping : String = _
@Description( """
Options used by LLVM clangpp
during *.cpp
sources compilation.
These options are added to auto-discovered LLVM options.
Sets standard by default.
Separator parameter: commonSequenceSeparator.
""" )
@Parameter(
property = "scalor.nativeOptionsClangPP",
defaultValue = "-std=c++11 ★"
)
var nativeOptionsClangPP : String = _
def nativeObjCopyMaps = parseCommonMapping( nativeObjcopyMapping )
def nativeOptsObjCopy = parseCommonList( nativeOptionsObjcopy )
def nativeOptsClangPP = parseCommonList( nativeOptionsClangPP )
def nativeOptsCompRelease = parseCommonList( nativeOptionsCompileRelease )
def nativeOptsCompDebug = parseCommonList( nativeOptionsCompileDebug )
def nativeOptsLinkRelease = parseCommonList( nativeOptionsLinkingRelease )
def nativeOptsLinkDebug = parseCommonList( nativeOptionsLinkingDebug )
}
trait ParamsLogging {
@Description( """
Enable logging of linker options.
Use to review actual Scala.native linker invocation configuration.
""" )
@Parameter(
property = "scalor.nativeLogOptions",
defaultValue = "false"
)
var nativeLogOptions : Boolean = _
@Description( """
Enable logging of Scala.native linker runtime.
Use to review actual generated output runtime
location.
""" )
@Parameter(
property = "scalor.nativeLogRuntime",
defaultValue = "false"
)
var nativeLogRuntime : Boolean = _
@Description( """
Enable logging of Scala.native linker class path.
Use to review actual resources used for *.nir
class discovery.
""" )
@Parameter(
property = "scalor.nativeLogClassPath",
defaultValue = "false"
)
var nativeLogClassPath : Boolean = _
@Description( """
Enable logging of Scala.native build results.
Use to review generated resources locations and counts.
""" )
@Parameter(
property = "scalor.nativeLogBuildStats",
defaultValue = "false"
)
var nativeLogBuildStats : Boolean = _
@Description( """
Enable logging of Scala.native build phase durations.
Use to review compiler and linker performance profile.
""" )
@Parameter(
property = "scalor.nativeLogBuildTimes",
defaultValue = "false"
)
var nativeLogBuildTimes : Boolean = _
@Description( """
Enable logging of Scala.native external executable invocations.
Use to review actual shell commands used to invoke external processes such as LLMV.
""" )
@Parameter(
property = "scalor.nativeLogBuildProcs",
defaultValue = "false"
)
var nativeLogBuildProcs : Boolean = _
@Description( """
Enable logging of Scala.native external command invocations "vertically".
Use to ease review of typical long LLVM compile and linking shell commands.
""" )
@Parameter(
property = "scalor.nativeLogBuildVerts",
defaultValue = "false"
)
var nativeLogBuildVerts : Boolean = _
@Description( """
Enable logging of Scala.native linker update result of M2E incremental change detection.
Use to review actual *.nir
classes which triggered Eclipse linker build.
""" )
@Parameter(
property = "scalor.nativeLogUpdateResult",
defaultValue = "false"
)
var nativeLogUpdateResult : Boolean = _
}
trait ParamsLibrary {
@Description( """
Regular expression used to discover Scala.native core *.nir
library from class path.
This regular expression is matched against resolved project depenencies in given scope.
Regular expression in the form: ${groupId}:${artifactId}
.
Enablement parameter: nativeLibraryDetect.
""" )
@Parameter(
property = "scalor.nativeScalaLibRegex",
defaultValue = "org.scala-native:scalalib_.+"
)
var nativeScalaLibRegex : String = _
@Description( """
Regular expression used to discover Scala.native interop C/CPP library from class path.
This library normally comes as dependency to scalalib
,
see nativeScalaLibRegex.
This regular expression is matched against resolved project depenencies in given scope.
Regular expression in the form: ${groupId}:${artifactId}
.
""" )
@Parameter(
property = "scalor.nativeNativeLibRegex",
defaultValue = "org.scala-native:nativelib_.+"
)
var nativeNativeLibRegex : String = _
@Description( """
Invoke Scala.native linker only when Scala.native library is detected
in project dependencies with given scope.
Detection parameter: nativeScalaLibRegex.
""" )
@Parameter(
property = "scalor.nativeLibraryDetect",
defaultValue = "true"
)
var nativeLibraryDetect : Boolean = _
}
trait ParamsRegex {
@Description( """
Regular expression used to discover Scala.native *.nir
IR classes from class path.
""" )
@Parameter(
property = "scalor.nativeClassRegex",
defaultValue = ".+[.]nir"
)
var nativeClassRegex : String = _
}
trait ParamsEntryClassAny {
def nativeEntryClass : String
}
trait ParamsEntryClassMain extends ParamsEntryClassAny {
@Description( """
Entry point for native runtime in scope=main.
Fully qualified class name which follows Java main
contract.
For example, Scala object in file main/Main.scala
:
package main
object Main {
def main( args : Array[ String ] ) : Unit = {
println( s"scala-native" )
}
}
""" )
@Parameter(
property = "scalor.nativeMainEntryClass",
defaultValue = "main.Main"
)
var nativeMainEntryClass : String = _
override def nativeEntryClass = nativeMainEntryClass
}
trait ParamsEntryClassTest extends ParamsEntryClassAny {
@Description( """
Entry point for native runtime in scope=test.
Fully qualified class name which follows Java main
contract.
For example, Scala object in file test/Main.scala
:
package test
object Main {
def main( args : Array[ String ] ) : Unit = {
println( s"scala-native" )
}
}
""" )
@Parameter(
property = "scalor.nativeTestEntryClass",
defaultValue = "test.Main"
)
var nativeTestEntryClass : String = _
override def nativeEntryClass = nativeTestEntryClass
}
trait ParamsGarbageCollectorAny {
def nativeGarbageCollector : String
}
trait ParamsGarbageCollectorMain extends ParamsGarbageCollectorAny {
@Description( """
Select garbage collector included with Scala.native runtime in scope=main.
Garbage collector reference.
Available garbage collectors:
none
boehm
immix
""" )
@Parameter(
property = "scalor.nativeMainGarbageCollector",
defaultValue = "immix"
)
var nativeMainGarbageCollector : String = _
override def nativeGarbageCollector = nativeMainGarbageCollector
}
trait ParamsGarbageCollectorTest extends ParamsGarbageCollectorAny {
@Description( """
Select garbage collector included with Scala.native runtime in scope=test.
Garbage collector reference.
Available garbage collectors:
none
boehm
immix
""" )
@Parameter(
property = "scalor.nativeTestGarbageCollector",
defaultValue = "boehm"
)
var nativeTestGarbageCollector : String = _
override def nativeGarbageCollector = nativeTestGarbageCollector
}
trait ParamsOperatingSystem {
import org.apache.commons.lang3.SystemUtils
@Description( """
Detect operating system and invoke native goals only when running on supported o/s.
When false
, force native goals invocation.
""" )
@Parameter(
property = "scalor.nativeSystemDetect",
defaultValue = "true"
)
var nativeSystemDetect : Boolean = _
/**
* http://www.scala-native.org/en/latest/user/setup.html
*/
def nativeHasOperatingSystem = {
import SystemUtils._
IS_OS_LINUX || IS_OS_MAC_OSX || IS_OS_FREE_BSD
}
}
trait ParamsLinkMain extends ParamsLinkAny
with BuildMain
with ParamsEntryClassMain
with ParamsGarbageCollectorMain {
@Description( """
Linker working directory for scope=main.
""" )
@Parameter(
property = "scalor.nativeMainWorkdir",
defaultValue = "${project.build.directory}/scalor/native/workdir/main"
)
var nativeMainWorkdir : File = _
override def nativeWorkdir = nativeMainWorkdir
}
trait ParamsLinkTest extends ParamsLinkAny
with BuildTest
with ParamsEntryClassTest
with ParamsGarbageCollectorTest {
@Description( """
Linker working directory for scope=main.
""" )
@Parameter(
property = "scalor.nativeTestWorkdir",
defaultValue = "${project.build.directory}/scalor/native/workdir/test"
)
var nativeTestWorkdir : File = _
override def nativeWorkdir = nativeTestWorkdir
}
trait ParamsPackAny extends AnyRef
with ParamsLibrary {
@Description( """
Configuration of Scala.native archive jar.
Normally used with provided default values.
Component reference:
MavenArchiveConfiguration
""" )
@Parameter()
var nativeArchiveConfig : MavenArchiveConfiguration = new MavenArchiveConfiguration()
@Description( """
Maven project helper.
""" )
@Component()
var projectHelper : MavenProjectHelper = _
// @Description( """
// Contains the full list of projects in the build.
// """ )
// @Parameter( defaultValue = "${reactorProjects}", readonly = true )
// var reactorProjects : java.util.List[ MavenProject ] = _
@Description( """
Root name for the generated Scala.native jar file.
Full name will include classifier
suffix.
""" )
@Parameter(
property = "scalor.nativeFinalName",
defaultValue = "${project.build.finalName}"
)
var nativeFinalName : String = _
def nativeHasAttach : Boolean
def nativeClassifier : String
def nativeOutputFolder : File
def nativeArchiveName = s"${nativeFinalName}-${nativeClassifier}.jar"
}
trait ParamsPackMain extends ParamsPackAny {
@Description( """
Artifact classifier for Scala.native with scope=main.
Appended to nativeFinalName.
""" )
@Parameter(
property = "scalor.nativeMainClassifier",
defaultValue = "native"
)
var nativeMainClassifier : String = _
@Description( """
Enable to attach generated Scala.native jar
to the project as deployment artifact with scope=main.
""" )
@Parameter(
property = "scalor.nativeMainAttach",
defaultValue = "true"
)
var nativeMainAttach : Boolean = _
@Description( """
Folder with generated Scala.native content with scope=main.
""" )
@Parameter(
property = "scalor.nativeMainOutputFolder",
defaultValue = "${project.build.directory}/scalor/native/output/main"
)
var nativeMainOutputFolder : File = _
override def nativeHasAttach = nativeMainAttach
override def nativeClassifier = nativeMainClassifier
override def nativeOutputFolder = nativeMainOutputFolder
}
trait ParamsPackTest extends ParamsPackAny {
@Description( """
Artifact classifier for Scala.native with scope=test.
Appended to nativeFinalName.
""" )
@Parameter(
property = "scalor.nativeTestClassifier",
defaultValue = "test-native"
)
var nativeTestClassifier : String = _
@Description( """
Enable to attach generated Scala.native jar
to the project as deployment artifact with scope=test.
""" )
@Parameter(
property = "scalor.nativeTestAttach",
defaultValue = "true"
)
var nativeTestAttach : Boolean = _
@Description( """
Folder with generated Scala.native content with scope=test.
""" )
@Parameter(
property = "scalor.nativeTestOutputFolder",
defaultValue = "${project.build.directory}/scalor/native/output/test"
)
var nativeTestOutputFolder : File = _
override def nativeHasAttach = nativeTestAttach
override def nativeClassifier = nativeTestClassifier
override def nativeOutputFolder = nativeTestOutputFolder
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy