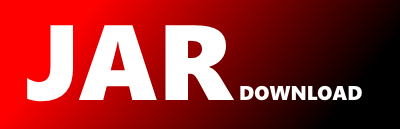
com.carrotsearch.lingo3g.integrations.elasticsearch.Lingo3gPlugin Maven / Gradle / Ivy
package com.carrotsearch.lingo3g.integrations.elasticsearch;
import com.carrotsearch.licensing.LicenseException;
import com.carrotsearch.lingo3g.Lingo3GClusteringAlgorithm;
import java.io.IOException;
import java.nio.file.Path;
import java.security.AccessController;
import java.security.PrivilegedAction;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.stream.Stream;
import org.carrot2.language.LanguageComponents;
import org.carrot2.language.LanguageComponentsLoader;
import org.carrot2.language.LanguageComponentsProvider;
import org.elasticsearch.client.Client;
import org.elasticsearch.cluster.metadata.IndexNameExpressionResolver;
import org.elasticsearch.cluster.service.ClusterService;
import org.elasticsearch.common.io.stream.NamedWriteableRegistry;
import org.elasticsearch.common.xcontent.NamedXContentRegistry;
import org.elasticsearch.env.Environment;
import org.elasticsearch.env.NodeEnvironment;
import org.elasticsearch.plugins.Plugin;
import org.elasticsearch.script.ScriptService;
import org.elasticsearch.threadpool.ThreadPool;
import org.elasticsearch.watcher.ResourceWatcherService;
import org.slf4j.LoggerFactory;
/**
* Elasticsearch extension plugin adding Lingo3G clustering algorithm support to elasticsearch-carrot2.
*/
public class Lingo3gPlugin extends Plugin {
public static final String PLUGIN_NAME = "elasticsearch-lingo3g";
@Override
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy