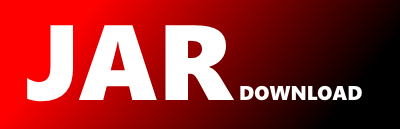
com.carrotsearch.hppc.LongDoubleMap Maven / Gradle / Ivy
package com.carrotsearch.hppc;
import com.carrotsearch.hppc.cursors.LongDoubleCursor;
/**
* An associative container with unique binding from keys to a single value.
*/
@javax.annotation.Generated(date = "2013-07-08T23:51:32+0200", value = "HPPC generated from: LongDoubleMap.java")
public interface LongDoubleMap
extends LongDoubleAssociativeContainer
{
/**
* Place a given key and value in the container.
*
* @return The value previously stored under the given key in the map is returned.
*/
public double put(long key, double value);
/**
* @return Returns the value associated with the given key or the default value
* for the key type, if the key is not associated with any value.
*
* Important note: For primitive type values, the value returned for a non-existing
* key may not be the default value of the primitive type (it may be any value previously
* assigned to that slot).
*/
public double get(long key);
/**
* Puts all keys from another container to this map, replacing the values
* of existing keys, if such keys are present.
*
* @return Returns the number of keys added to the map as a result of this
* call (not previously present in the map). Values of existing keys are overwritten.
*/
public int putAll(
LongDoubleAssociativeContainer container);
/**
* Puts all keys from an iterable cursor to this map, replacing the values
* of existing keys, if such keys are present.
*
* @return Returns the number of keys added to the map as a result of this
* call (not previously present in the map). Values of existing keys are overwritten.
*/
public int putAll(
Iterable extends LongDoubleCursor> iterable);
/**
* Remove all values at the given key. The default value for the key type is returned
* if the value does not exist in the map.
*/
public double remove(long key);
/**
* Compares the specified object with this set for equality. Returns
* true if and only if the specified object is also a
* {@link LongDoubleMap} and both objects contains exactly the same key-value pairs.
*/
public boolean equals(Object obj);
/**
* @return A hash code of elements stored in the map. The hash code
* is defined as a sum of hash codes of keys and values stored
* within the set). Because sum is commutative, this ensures that different order
* of elements in a set does not affect the hash code.
*/
public int hashCode();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy