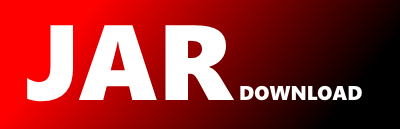
com.carrotsearch.hppc.LongStack Maven / Gradle / Ivy
Go to download
High Performance Primitive Collections: data structures (maps, sets, lists, stacks, queues) generated for combinations of object and primitive types to conserve JVM memory and speed up execution.
The newest version!
package com.carrotsearch.hppc;
/* */
import com.carrotsearch.hppc.cursors.LongCursor;
/**
* A subclass of {@link LongArrayList} adding stack-related utility methods.
* The top of the stack is at the {@link #size()} - 1
element.
*/
@com.carrotsearch.hppc.Generated(
date = "2018-05-21T12:24:05+0200",
value = "KTypeStack.java")
public class LongStack extends LongArrayList {
/**
* New instance with sane defaults.
*/
public LongStack() {
super();
}
/**
* New instance with sane defaults.
*
* @param expectedElements
* The expected number of elements guaranteed not to cause buffer
* expansion (inclusive).
*/
public LongStack(int expectedElements) {
super(expectedElements);
}
/**
* New instance with sane defaults.
*
* @param expectedElements
* The expected number of elements guaranteed not to cause buffer
* expansion (inclusive).
* @param resizer
* Underlying buffer sizing strategy.
*/
public LongStack(int expectedElements, ArraySizingStrategy resizer) {
super(expectedElements, resizer);
}
/**
* Create a stack by pushing all elements of another container to it.
*/
public LongStack(LongContainer container) {
super(container);
}
/**
* Adds one long to the stack.
*/
public void push(long e1) {
ensureBufferSpace(1);
buffer[elementsCount++] = e1;
}
/**
* Adds two longs to the stack.
*/
public void push(long e1, long e2) {
ensureBufferSpace(2);
buffer[elementsCount++] = e1;
buffer[elementsCount++] = e2;
}
/**
* Adds three longs to the stack.
*/
public void push(long e1, long e2, long e3) {
ensureBufferSpace(3);
buffer[elementsCount++] = e1;
buffer[elementsCount++] = e2;
buffer[elementsCount++] = e3;
}
/**
* Adds four longs to the stack.
*/
public void push(long e1, long e2, long e3, long e4) {
ensureBufferSpace(4);
buffer[elementsCount++] = e1;
buffer[elementsCount++] = e2;
buffer[elementsCount++] = e3;
buffer[elementsCount++] = e4;
}
/**
* Add a range of array elements to the stack.
*/
public void push(long[] elements, int start, int len) {
assert start >= 0 && len >= 0;
ensureBufferSpace(len);
System.arraycopy(elements, start, buffer, elementsCount, len);
elementsCount += len;
}
/**
* Vararg-signature method for pushing elements at the top of the stack.
*
* This method is handy, but costly if used in tight loops (anonymous array
* passing)
*
*/
/* */
public final void push(long... elements) {
push(elements, 0, elements.length);
}
/**
* Pushes all elements from another container to the top of the stack.
*/
public int pushAll(LongContainer container) {
return addAll(container);
}
/**
* Pushes all elements from another iterable to the top of the stack.
*/
public int pushAll(Iterable extends LongCursor> iterable) {
return addAll(iterable);
}
/**
* Discard an arbitrary number of elements from the top of the stack.
*/
public void discard(int count) {
assert elementsCount >= count;
elementsCount -= count;
/* */
}
/**
* Discard the top element from the stack.
*/
public void discard() {
assert elementsCount > 0;
elementsCount--;
/* */
}
/**
* Remove the top element from the stack and return it.
*/
public long pop() {
assert elementsCount > 0;
final long v = buffer[--elementsCount];
/* */
return v;
}
/**
* Peek at the top element on the stack.
*/
public long peek() {
assert elementsCount > 0;
return buffer[elementsCount - 1];
}
/**
* Create a stack by pushing a variable number of arguments to it.
*/
/* */
public static LongStack from(long... elements) {
final LongStack stack = new LongStack(elements.length);
stack.push(elements);
return stack;
}
/**
* {@inheritDoc}
*/
@Override
public LongStack clone() {
return (LongStack) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy