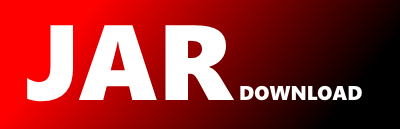
com.caucho.config.inject.AbstractSingletonBean Maven / Gradle / Ivy
/*
* Copyright (c) 1998-2018 Caucho Technology -- all rights reserved
*
* This file is part of Resin(R) Open Source
*
* Each copy or derived work must preserve the copyright notice and this
* notice unmodified.
*
* Resin Open Source is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* Resin Open Source is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE, or any warranty
* of NON-INFRINGEMENT. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License
* along with Resin Open Source; if not, write to the
*
* Free Software Foundation, Inc.
* 59 Temple Place, Suite 330
* Boston, MA 02111-1307 USA
*
* @author Scott Ferguson
*/
package com.caucho.config.inject;
import java.io.Closeable;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
import java.util.Set;
import javax.enterprise.context.spi.CreationalContext;
import javax.enterprise.inject.spi.Annotated;
import javax.enterprise.inject.spi.AnnotatedMethod;
import javax.enterprise.inject.spi.AnnotatedType;
import javax.enterprise.inject.spi.PassivationCapable;
import com.caucho.config.event.EventManager;
/**
* SingletonBean represents a singleton instance exported as a web beans.
*
*
* @Current Manager manager;
*
* manager.addBean(new SingletonBean(myValue));
*
*/
abstract public class AbstractSingletonBean extends BeanWrapper
implements Closeable, AnnotatedBean, PassivationCapable
{
private ManagedBeanImpl _managedBean;
private Set _types;
private Annotated _annotated;
private Set _qualifiers;
private Set> _stereotypes;
private Class extends Annotation> _scopeType;
private String _name;
private String _passivationId;
AbstractSingletonBean(ManagedBeanImpl managedBean,
Set types,
Annotated annotated,
Set bindings,
Set> stereotypes,
Class extends Annotation> scopeType,
String name)
{
super(managedBean.getBeanManager(), managedBean);
_managedBean = managedBean;
_types = types;
_annotated = annotated;
_qualifiers = bindings;
_stereotypes = stereotypes;
_scopeType = scopeType;
_name = name;
// ioc/0e13
_managedBean.setPassivationId(getId());
}
//
// metadata for the bean
//
@Override
public Annotated getAnnotated()
{
if (_annotated != null)
return _annotated;
else
return _managedBean.getAnnotated();
}
@Override
public AnnotatedType getAnnotatedType()
{
if (_annotated instanceof AnnotatedType>)
return (AnnotatedType) _annotated;
else
return _managedBean.getAnnotatedType();
}
@Override
public Set getQualifiers()
{
if (_qualifiers != null)
return _qualifiers;
else
return super.getQualifiers();
}
@Override
public Set> getStereotypes()
{
if (_stereotypes != null)
return _stereotypes;
else
return getBean().getStereotypes();
}
@Override
public String getName()
{
if (_name != null)
return _name;
else
return getBean().getName();
}
/**
* Return passivation id
*/
@Override
public String getId()
{
if (_passivationId == null)
_passivationId = calculatePassivationId();
return _passivationId;
}
public void introspectProduces()
{
ProducesBuilder builder = new ManagedProducesBuilder(getBeanManager());
builder.introspectProduces(this, getAnnotatedType());
}
public void introspectObservers()
{
EventManager eventManager = getBeanManager().getEventManager();
AnnotatedType annType = getAnnotatedType();
// ioc/0b25
for (AnnotatedMethod super T> beanMethod : annType.getMethods()) {
int param = EventManager.findObserverAnnotation(beanMethod);
if (param < 0)
continue;
// ioc/0b25
eventManager.addObserver(this, beanMethod);
}
}
/**
* Returns the bean's scope type.
*/
@Override
public Class extends Annotation> getScope()
{
if (_scopeType != null)
return _scopeType;
else
return getBean().getScope();
}
/**
* Returns the types that the bean exports for bindings.
*/
@Override
public Set getTypes()
{
if (_types != null)
return _types;
else
return getBean().getTypes();
}
@Override
abstract public T create(CreationalContext env);
/**
* Frees the singleton on environment shutdown
*/
@Override
public void close()
{
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy