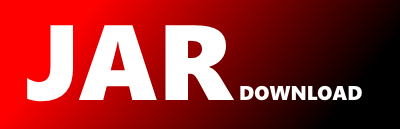
com.caucho.ejb.inject.SessionRegistrationBean Maven / Gradle / Ivy
/*
* Copyright (c) 1998-2018 Caucho Technology -- all rights reserved
*
* This file is part of Resin(R) Open Source
*
* Each copy or derived work must preserve the copyright notice and this
* notice unmodified.
*
* Resin Open Source is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* Resin Open Source is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE, or any warranty
* of NON-INFRINGEMENT. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License
* along with Resin Open Source; if not, write to the
*
* Free Software Foundation, Inc.
* 59 Temple Place, Suite 330
* Boston, MA 02111-1307 USA
*
* @author Scott Ferguson
*/
package com.caucho.ejb.inject;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
import java.util.HashSet;
import java.util.Set;
import javax.enterprise.context.spi.CreationalContext;
import javax.enterprise.inject.spi.Bean;
import com.caucho.config.inject.BeanAdapter;
import com.caucho.config.inject.CreationalContextImpl;
import com.caucho.config.inject.InjectEnvironmentBean;
import com.caucho.config.inject.InjectManager;
import com.caucho.config.j2ee.BeanName;
import com.caucho.config.j2ee.EjbQualifierLiteral;
import com.caucho.ejb.session.AbstractSessionContext;
import com.caucho.inject.Module;
/**
* Internal registration for the EJB.
*/
@Module
public class SessionRegistrationBean extends BeanAdapter
implements InjectEnvironmentBean
{
private AbstractSessionContext _context;
private Set _qualifierSet;
private Set _types;
public SessionRegistrationBean(InjectManager beanManager,
AbstractSessionContext context,
Bean bean,
BeanName beanName)
{
super(beanManager, bean);
_context = context;
_qualifierSet = new HashSet();
_qualifierSet.add(beanName);
_qualifierSet.add(EjbQualifierLiteral.QUALIFIER);
}
public InjectManager getCdiManager()
{
return _context.getInjectManager();
}
/**
* The registration bean is not registered by name
*/
@Override
public String getName()
{
return null;
}
@Override
public Set getQualifiers()
{
return _qualifierSet;
}
@Override
public T create(CreationalContext env)
{
T proxy;
if (env instanceof CreationalContextImpl>)
proxy = _context.createProxy((CreationalContextImpl) env);
else
proxy = _context.createProxy(null);
return proxy;
}
@Override
public void destroy(T instance, CreationalContext cxt)
{
CreationalContextImpl env;
if (cxt instanceof CreationalContextImpl>)
env = (CreationalContextImpl) cxt;
else
env = null;
_context.destroyProxy(instance, env);
}
@Override
public Set getTypes()
{
if (_types == null) {
_types = new HashSet();
// ejb/2018
_types.add(_context.getApi());
}
return _types;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy