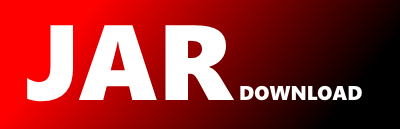
com.cedarsoft.spring.rcp.hierarchy.DetailsPanel Maven / Gradle / Ivy
The newest version!
package com.cedarsoft.spring.rcp.hierarchy;
import com.cedarsoft.spring.SpringSupport;
import org.jetbrains.annotations.NonNls;
import org.jetbrains.annotations.NotNull;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationEvent;
import org.springframework.richclient.application.Application;
import org.springframework.richclient.application.ApplicationServices;
import org.springframework.richclient.factory.ComponentFactory;
import javax.swing.JPanel;
import javax.swing.JSeparator;
import java.awt.BorderLayout;
/**
*
*/
public final class DetailsPanel extends JPanel {
public DetailsPanel( @NotNull HierarchyDetailsModel model ) {
super( new BorderLayout() );
JPanel titlePaneContainer = new JPanel( new BorderLayout() );
titlePaneContainer.add( model.getTopPanel() );
titlePaneContainer.add( new JSeparator(), BorderLayout.SOUTH );
add( BorderLayout.NORTH, titlePaneContainer );
add( BorderLayout.CENTER, model.getContentPanel() );
}
//Spring Support delegations
public String getMessage( @NotNull @NonNls String messageCode ) {
return SpringSupport.INSTANCE.getMessage( messageCode );
}
public ComponentFactory getComponentFactory() {
return SpringSupport.INSTANCE.getComponentFactory();
}
public S getService( Class serviceType ) {
return SpringSupport.INSTANCE.getService( serviceType );
}
public ApplicationServices getApplicationServices() {
return SpringSupport.INSTANCE.getApplicationServices();
}
public String getMessage( @NotNull @NonNls String messageCode, Object... objects ) {
return SpringSupport.INSTANCE.getMessage( messageCode, objects );
}
public Application getApplication() {
return SpringSupport.INSTANCE.getApplication();
}
public ApplicationContext getApplicationContext() {
return SpringSupport.INSTANCE.getApplicationContext();
}
public void publishEvent( @NotNull ApplicationEvent event ) {
SpringSupport.INSTANCE.publishEvent( event );
}
public void publishCreated( @NotNull Object object ) {
SpringSupport.INSTANCE.publishCreated( object );
}
public void publishDeleted( @NotNull Object object ) {
SpringSupport.INSTANCE.publishDeleted( object );
}
public void publishModified( @NotNull Object object ) {
SpringSupport.INSTANCE.publishModified( object );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy