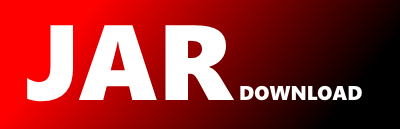
com.cedarsoft.spring.rcp.tree.layered.aspects.EditAspect Maven / Gradle / Ivy
package com.cedarsoft.spring.rcp.tree.layered.aspects;
import com.cedarsoft.spring.rcp.commands.CommandIds;
import com.cedarsoft.spring.rcp.tree.layered.DefaultTreeLayer;
import com.cedarsoft.spring.rcp.tree.layered.config.PopupConfigurer;
import org.jetbrains.annotations.NotNull;
import org.springframework.richclient.command.ActionCommand;
import javax.swing.JTree;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
*
*/
public class EditAspect implements TreeLayerAspect
{
@NotNull
private final Editor
editor;
public EditAspect( @NotNull Editor
editor ) {
this.editor = editor;
}
@Override
public void apply( @NotNull DefaultTreeLayer
layer ) {
layer.addPopupConfigurer( new PopupConfigurer
() {
@Override
protected List extends ActionCommand> getCommands( @NotNull final P parent, @NotNull final C selectedChild, @NotNull final JTree source ) {
List commands = new ArrayList();
ActionCommand editCommand = new ActionCommand( CommandIds.EDIT ) {
@Override
protected void doExecuteCommand() {
editor.editEntry( parent, selectedChild, source );
}
};
return Collections.singletonList( editCommand );
}
} );
}
/**
* Edits an entry
*
* @param the parent type
* @param the child type
*/
public interface Editor {
/**
* Edits an object
*
* @param parent the parent
* @param selectedChild the selected child
* @param source the source tree
*/
void editEntry( @NotNull P parent, @NotNull C selectedChild, @NotNull JTree source );
}
}