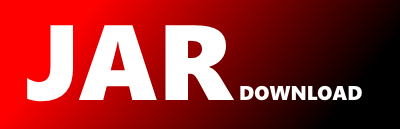
eu.cedarsoft.utils.springrcp.beanlist.ParentAwareBeanListView Maven / Gradle / Ivy
package com.cedarsoft.utils.springrcp.beanlist;
import com.cedarsoft.utils.springrcp.selection.Selection;
import com.cedarsoft.utils.springrcp.selection.SelectionManager;
import org.jetbrains.annotations.NotNull;
import org.springframework.richclient.command.CommandGroup;
import javax.swing.table.TableModel;
/**
* Shows a bean list view that is dependent on the selection of a "parent" bean
*
*
* T is the type of the beans that are shown within this view.
* P is the type of the "parent" bean.
*
* Date: 24.08.2006
* Time: 18:02:25
*
* @author Johannes Schneider -
* Xore Systems
*/
public class ParentAwareBeanListView extends BeanListView {
private Class parentType;
@Override
protected void initSelectionChanges() {
super.initSelectionChanges();
//When the parent bean changes --> nearly everything should be updated
SelectionManager.WindowSelectionManager windowSelectionManager = SelectionManager.getInstance().getWindowSelectionManager( getContext().getWindow() );
windowSelectionManager.addSelectionListener( parentType, new SelectionManager.SelectionListener
() {
public void notifySelectionChanged( @NotNull Selection
selection ) {
updateParentAwareElements( selection );
}
} );
}
@Override
protected void finalizeInitialization() {
SelectionManager.WindowSelectionManager windowSelectionManager = SelectionManager.getInstance().getWindowSelectionManager( getContext().getWindow() );
Selection
selection = windowSelectionManager.getSelection( getParentType() );
updateParentAwareElements( selection );
}
@NotNull
@Override
protected CommandGroup createDefaultCommandGroup() {
getCommandFactory().getNewCommand().setEnabled( false );
return super.createDefaultCommandGroup();
}
protected void updateParentAwareElements( @NotNull Selection
selection ) {
/**
* (dis/en)able new command
*/
getCommandFactory().getNewCommand().setEnabled( !selection.isEmty() );
TableModel tableModel = getTableModel();
if ( tableModel instanceof ParentAware ) {
ParentAware
parentAware = ( ParentAware ) tableModel;
if ( selection.isEmty() ) {
parentAware.setParent( null );
}
if ( selection.isSingleSelection() ) {
parentAware.setParent( parentAware.getParentType().cast( selection.getSingleSelection() ) );
}
if ( selection.isMultiSelection() ) {
throw new UnsupportedOperationException( "not implemented for multi selection" );
}
}
}
@NotNull
public Class
getParentType() {
if ( parentType == null ) {
throw new IllegalStateException( "No ParentType has been set" );
}
return parentType;
}
public void setParentType( @NotNull Class
parentType ) {
this.parentType = parentType;
}
}