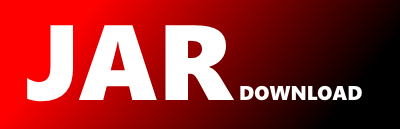
com.cedarsoft.gdao.DefaultGenericServiceManager Maven / Gradle / Ivy
package com.cedarsoft.gdao;
import com.cedarsoft.utils.Cache;
import com.cedarsoft.utils.HashedCache;
import org.jetbrains.annotations.NotNull;
import org.springframework.transaction.support.AbstractPlatformTransactionManager;
/**
* Manages the services
*/
public class DefaultGenericServiceManager implements GenericServiceManager {
@NotNull
private final GenericDaoManager daoManager;
@NotNull
private final AbstractPlatformTransactionManager transactionManager;
@SuppressWarnings( {"MismatchedQueryAndUpdateOfCollection"} )
@NotNull
private final Cache, GenericService
© 2015 - 2025 Weber Informatics LLC | Privacy Policy