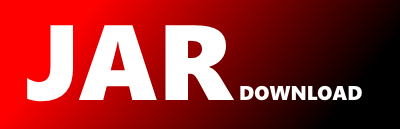
com.cedarsoft.gdao.GenericService Maven / Gradle / Ivy
package com.cedarsoft.gdao;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
/**
* A generic service that extends the behaviour of simple DAOs with transaction support.
*/
public interface GenericService extends GenericDao {
/**
* Performs an action within an transaction.
*
* @param callback the callback that is called within a transaction
* @return the return value (optional)
*/
@Nullable
R perform( @NotNull ServiceCallback callback );
/**
* A callback that can be used to be invoked within a service (in a transaction).
*/
interface ServiceCallback {
/**
* Perform the action
*
* @param service the service
* @return the return value (may be null)
*/
@Nullable
R perform( @NotNull GenericService service );
}
/**
* Abstract base class for service callbacks without return value.
*/
abstract class ServiceCallbackWithoutReturnValue implements ServiceCallback {
@Nullable
public Object perform( @NotNull GenericService service ) {
performVoid( service );
return null;
}
/**
* Perform the action without any return value
*
* @param service the service
*/
protected abstract void performVoid( @NotNull GenericService service );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy