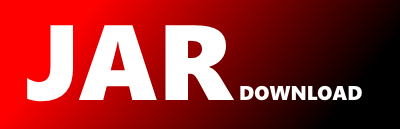
com.cedarsoftware.util.ProxyFactory Maven / Gradle / Ivy
package com.cedarsoftware.util;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Proxy;
/**
* Created by kpartlow on 4/30/2014.
*/
public final class ProxyFactory
{
/**
* This class should be used statically
*/
private ProxyFactory() {}
/**
* Returns an instance of a proxy class for the specified interfaces
* that dispatches method invocations to the specified invocation
* handler.
*
* @param intf the interface for the proxy to implement
* @param h the invocation handler to dispatch method invocations to
* @return a proxy instance with the specified invocation handler of a
* proxy class that is defined by the specified class loader
* and that implements the specified interfaces
* @throws IllegalArgumentException if any of the restrictions on the
* parameters that may be passed to getProxyClass
* are violated
* @throws NullPointerException if the interfaces
array
* argument or any of its elements are null
, or
* if the invocation handler, h
, is
* null
*/
public static T create(Class intf, InvocationHandler h) {
return create(h.getClass().getClassLoader(), intf, h);
}
/**
* Returns an instance of a proxy class for the specified interfaces
* that dispatches method invocations to the specified invocation
* handler.
*
* @param loader the class loader to define the proxy class
* @param intf the interface for the proxy to implement
* @param h the invocation handler to dispatch method invocations to
* @return a proxy instance with the specified invocation handler of a
* proxy class that is defined by the specified class loader
* and that implements the specified interfaces
* @throws IllegalArgumentException if any of the restrictions on the
* parameters that may be passed to getProxyClass
* are violated
* @throws NullPointerException if the interfaces
array
* argument or any of its elements are null
, or
* if the invocation handler, h
, is
* null
*/
public static T create(ClassLoader loader, Class intf, InvocationHandler h) {
return (T)Proxy.newProxyInstance(loader, new Class[]{intf}, h);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy