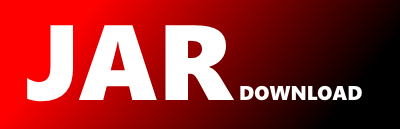
com.cedarsoftware.util.ArrayUtilities Maven / Gradle / Ivy
package com.cedarsoftware.util;
import java.lang.reflect.Array;
import java.util.Arrays;
import java.util.Collection;
import java.util.Iterator;
/**
* Handy utilities for working with Java arrays.
*
* @author Ken Partlow
* @author John DeRegnaucourt ([email protected])
*
* Copyright (c) Cedar Software LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* License
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
public final class ArrayUtilities
{
/**
* Immutable common arrays.
*/
public static final Object[] EMPTY_OBJECT_ARRAY = new Object[0];
public static final byte[] EMPTY_BYTE_ARRAY = new byte[0];
public static final char[] EMPTY_CHAR_ARRAY = new char[0];
public static final Character[] EMPTY_CHARACTER_ARRAY = new Character[0];
public static final Class[] EMPTY_CLASS_ARRAY = new Class[0];
/**
* Private constructor to promote using as static class.
*/
private ArrayUtilities()
{
super();
}
/**
* This is a null-safe isEmpty check. It uses the Array
* static class for doing a length check. This check is actually
* .0001 ms slower than the following typed check:
*
* return array == null || array.length == 0;
*
* but gives you more flexibility, since it checks for all array
* types.
*
* @param array array to check
* @return true if empty or null
*/
public static boolean isEmpty(final Object array)
{
return array == null || Array.getLength(array) == 0;
}
/**
* This is a null-safe size check. It uses the Array
* static class for doing a length check. This check is actually
* .0001 ms slower than the following typed check:
*
* return (array == null) ? 0 : array.length;
*
* @param array array to check
* @return true if empty or null
*/
public static int size(final Object array)
{
return array == null ? 0 : Array.getLength(array);
}
/**
* Shallow copies an array of Objects
*
* The objects in the array are not cloned, thus there is no special
* handling for multi-dimensional arrays.
*
* This method returns null
if null
array input.
*
* @param array the array to shallow clone, may be null
* @param the array type
* @return the cloned array, null
if null
input
*/
public static T[] shallowCopy(final T[] array)
{
if (array == null)
{
return null;
}
return array.clone();
}
/**
* Adds all the elements of the given arrays into a new array.
*
* The new array contains all of the element of array1
followed
* by all of the elements array2
. When an array is returned, it is always
* a new array.
*
*
* ArrayUtilities.addAll(null, null) = null
* ArrayUtilities.addAll(array1, null) = cloned copy of array1
* ArrayUtilities.addAll(null, array2) = cloned copy of array2
* ArrayUtilities.addAll([], []) = []
* ArrayUtilities.addAll([null], [null]) = [null, null]
* ArrayUtilities.addAll(["a", "b", "c"], ["1", "2", "3"]) = ["a", "b", "c", "1", "2", "3"]
*
*
* @param array1 the first array whose elements are added to the new array, may be null
* @param array2 the second array whose elements are added to the new array, may be null
* @param the array type
* @return The new array, null
if null
array inputs.
* The type of the new array is the type of the first array.
*/
@SuppressWarnings("unchecked")
public static T[] addAll(final T[] array1, final T[] array2)
{
if (array1 == null)
{
return shallowCopy(array2);
}
else if (array2 == null)
{
return shallowCopy(array1);
}
final T[] newArray = (T[]) Array.newInstance(array1.getClass().getComponentType(), array1.length + array2.length);
System.arraycopy(array1, 0, newArray, 0, array1.length);
System.arraycopy(array2, 0, newArray, array1.length, array2.length);
return newArray;
}
@SuppressWarnings("unchecked")
public static T[] removeItem(T[] array, int pos)
{
final int len = Array.getLength(array);
T[] dest = (T[]) Array.newInstance(array.getClass().getComponentType(), len - 1);
System.arraycopy(array, 0, dest, 0, pos);
System.arraycopy(array, pos + 1, dest, pos, len - pos - 1);
return dest;
}
public static T[] getArraySubset(T[] array, int start, int end)
{
return Arrays.copyOfRange(array, start, end);
}
/**
* Convert Collection to a Java (typed) array [].
* @param classToCastTo array type (Object[], Person[], etc.)
* @param c Collection containing items to be placed into the array.
* @param Type of the array
* @return Array of the type (T) containing the items from collection 'c'.
*/
@SuppressWarnings("unchecked")
public static T[] toArray(Class classToCastTo, Collection> c)
{
T[] array = c.toArray((T[]) Array.newInstance(classToCastTo, c.size()));
Iterator i = c.iterator();
int idx = 0;
while (i.hasNext())
{
Array.set(array, idx++, i.next());
}
return array;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy