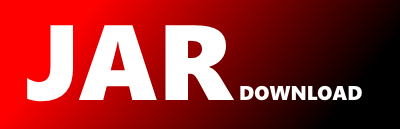
com.cedarsoftware.ncube.RuleInfo.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of n-cube Show documentation
Show all versions of n-cube Show documentation
Multi-dimensional Rule Engine
package com.cedarsoftware.ncube
import com.cedarsoftware.util.CaseInsensitiveMap
import com.cedarsoftware.util.CaseInsensitiveSet
import com.cedarsoftware.util.CompactCILinkedMap
import groovy.transform.CompileStatic
/**
* This class contains information about the rule execution.
*
* @author John DeRegnaucourt ([email protected])
*
* Copyright (c) Cedar Software LLC
*
* Licensed under the Apache License, Version 2.0 (the "License")
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
@CompileStatic
class RuleInfo extends CaseInsensitiveMap
{
// Convert Enums to String constants for performance gain
public static final String RULES_EXECUTED = 'RULES_EXECUTED'
public static final String RULE_STOP = 'RULE_STOP'
public static final String SYSTEM_OUT = 'SYSTEM_OUT'
public static final String SYSTEM_ERR = 'SYSTEM_ERR'
public static final String ASSERTION_FAILURES = 'ASSERTION_FAILURES'
public static final String LAST_EXECUTED_STATEMENT = 'LAST_EXECUTED_STATEMENT'
public static final String AXIS_BINDINGS = 'AXIS_BINDINGS'
public static final String INPUT_KEYS_USED = 'INPUT_KEYS_ACCESSED'
public static final String UNBOUND_AXES_USED = 'UNBOUND_AXES_ACCESSED'
RuleInfo()
{
// For speed, we are using the String (no function call - this code gets executed frequently)
// Key = RuleMetaKeys.RULES_EXECUTED.name()
put(RULES_EXECUTED, [])
}
/**
* @return long indicating the number of conditions that fired (and therefore steps that executed).
*/
long getNumberOfRulesExecuted()
{
return axisBindings.size()
}
/**
* Set the indicator that a ruleStop was thrown
*/
protected void ruleStopThrown()
{
put(RULE_STOP, Boolean.TRUE)
}
/**
* @return true if a RuleStop was thrown during rule execution
*/
boolean wasRuleStopThrown()
{
return containsKey(RULE_STOP) && Boolean.TRUE == get(RULE_STOP)
}
/**
* @return String output of all println calls that occurred from the start of the initial getCell() call
* until it returned.
*/
String getSystemOut()
{
if (containsKey(SYSTEM_OUT))
{
return (String) get(SYSTEM_OUT)
}
return ''
}
void setSystemOut(String out)
{
put(SYSTEM_OUT, out)
}
/**
* @return String output of system.err that occurred from the start of the initial getCell() call
* until it returned. Kept separately per thread.
*/
String getSystemErr()
{
if (containsKey(SYSTEM_ERR))
{
return (String) get(SYSTEM_ERR)
}
return ''
}
void setSystemErr(String err)
{
put(SYSTEM_ERR, err)
}
/**
* @return Set of String assert failures that occurred when runTest was called.
*/
Set getAssertionFailures()
{
if (containsKey(ASSERTION_FAILURES))
{
return (Set) get(ASSERTION_FAILURES)
}
Set failures = new CaseInsensitiveSet<>()
put(ASSERTION_FAILURES, failures)
return failures
}
void setAssertionFailures(Set failures)
{
put(ASSERTION_FAILURES, failures)
}
/**
* @return Object value of last executed statement value.
*/
Object getLastExecutedStatementValue()
{
if (containsKey(LAST_EXECUTED_STATEMENT))
{
return get(LAST_EXECUTED_STATEMENT)
}
return null
}
protected void setLastExecutedStatement(Object value)
{
put(LAST_EXECUTED_STATEMENT, value)
}
/**
* @return List of Binding instances which describe each Binding per getCell() call. A binding
* holds the axisNames and values that were supplied.
*/
List getAxisBindings()
{
if (containsKey(AXIS_BINDINGS))
{
return (List)get(AXIS_BINDINGS)
}
List bindings = []
put(AXIS_BINDINGS, bindings)
return bindings
}
/**
* @return Set of input keys that were used (.get() or .containsKey() accessed) from the input Map.
*/
Set getInputKeysUsed()
{
Set keysUsed = (Set)get(INPUT_KEYS_USED)
if (keysUsed == null)
{
keysUsed = new CaseInsensitiveSet(Collections.emptyList(), new CaseInsensitiveMap(Collections.emptyMap(), new HashMap<>()))
put(INPUT_KEYS_USED, keysUsed)
}
return keysUsed
}
protected void addInputKeysUsed(Collection keys)
{
inputKeysUsed.addAll(keys)
}
/**
* @return List of map entries where the key is a cube name and the value is
* another map entry. The second map entry contains the name of an
* unbound axis and the value that could not be bound.
*/
List getUnboundAxesList()
{
List unBoundAxesList = get(UNBOUND_AXES_USED) as List
if (unBoundAxesList == null)
{
unBoundAxesList = []
put(UNBOUND_AXES_USED, unBoundAxesList)
}
return unBoundAxesList
}
/**
* @return Map of cube names containing a map keyed by axis name.
* Each axis name map contains a list of column values that could
* not be bound.
*/
Map>> getUnboundAxesMap()
{
Map>> unBoundAxesMap = new CompactCILinkedMap<>()
unboundAxesList.each { MapEntry entry ->
String cubeName = entry.key
MapEntry axisBinding = entry.value as MapEntry
String axisName = axisBinding.key
Map> axisMap = unBoundAxesMap.get(cubeName)
if (axisMap == null)
{
axisMap = new CompactCILinkedMap<>()
unBoundAxesMap.put(cubeName, axisMap)
}
Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy