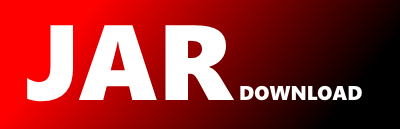
com.cedarsoftware.ncube.formatters.TestResultsFormatter.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of n-cube Show documentation
Show all versions of n-cube Show documentation
Multi-dimensional Rule Engine
package com.cedarsoftware.ncube.formatters
import com.cedarsoftware.ncube.Binding
import com.cedarsoftware.ncube.NCube
import com.cedarsoftware.ncube.RuleInfo
import groovy.transform.CompileStatic
/**
* Format test result output for display in HTML. This includes
* showing the last executed statement value, execution path,
* assertion results, output map, System.out, and System.err.
*
* @author Ken Partlow ([email protected])
*
* Copyright (c) Cedar Software LLC
*
* Licensed under the Apache License, Version 2.0 (the "License")
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
@CompileStatic
class TestResultsFormatter
{
private final Map output
private final StringBuilder builder = new StringBuilder()
private static final String newLine = '\n'
TestResultsFormatter(Map out)
{
this.output = out
}
String format()
{
formatLastExecutedStatement()
formatAxisBinding()
formatAssertions()
formatOutputMap()
formatSystemOut("System.out")
formatSystemOut("System.err")
return builder.toString()
}
private void formatLastExecutedStatement()
{
RuleInfo ruleInfo = (RuleInfo) output.get(NCube.RULE_EXEC_INFO)
builder.append("Last statement (cell) executed")
builder.append("")
builder.append(newLine)
builder.append(ruleInfo.getLastExecutedStatementValue())
builder.append(newLine)
builder.append("
")
}
private void formatAxisBinding()
{
RuleInfo ruleInfo = (RuleInfo) output.get(NCube.RULE_EXEC_INFO)
builder.append("Execution flow")
builder.append("")
builder.append(newLine)
for (Iterator iterator = ruleInfo.getAxisBindings().iterator(); iterator.hasNext(); )
{
Binding binding = iterator.next()
builder.append(binding.toHtml())
builder.append(newLine)
if (iterator.hasNext())
{
builder.append("
")
}
}
builder.append("
")
}
private void formatAssertions()
{
RuleInfo ruleInfo = (RuleInfo) output.get(NCube.RULE_EXEC_INFO)
builder.append("Assertions")
builder.append("")
builder.append(newLine)
Set failures = ruleInfo.getAssertionFailures()
if (failures.empty)
{
builder.append("No assertion failures")
builder.append(newLine)
}
else
{
for (String entry : failures)
{
builder.append(entry)
builder.append(newLine)
}
builder.length = builder.length()-1
}
builder.append("
")
}
private void formatOutputMap()
{
builder.append("Output Map")
builder.append("")
builder.append(newLine)
if (output.containsKey(NCube.RULE_EXEC_INFO) && output.containsKey("return") && output.size() <= 2)
{ // size() == 1 minimum (_rule metakey).
builder.append("No output")
builder.append(newLine)
}
else
{
for (item in output.entrySet())
{
Object key = item.key
if (NCube.RULE_EXEC_INFO.equals(key))
{
continue
}
builder.append(key)
builder.append(" = ")
builder.append(item.value)
builder.append(newLine)
}
}
builder.append("
")
}
private void formatSystemOut(String section)
{
boolean isErr = section.toLowerCase().contains("err")
builder.append("")
builder.append(section)
builder.append("")
if (isErr)
{
builder.append("")
}
else
{
builder.append("")
}
builder.append(newLine)
RuleInfo ruleInfo = (RuleInfo) output.get(NCube.RULE_EXEC_INFO)
if (isErr)
{
builder.append(ruleInfo.getSystemErr())
}
else
{
builder.append(ruleInfo.getSystemOut())
}
builder.append("
")
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy