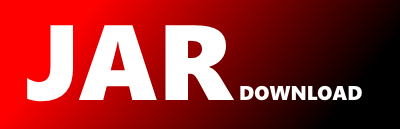
com.cedarsoftware.ncube.rules.BusinessRule.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of n-cube Show documentation
Show all versions of n-cube Show documentation
Multi-dimensional Rule Engine
package com.cedarsoftware.ncube.rules
import com.cedarsoftware.ncube.ApplicationID
import com.cedarsoftware.ncube.NCube
import com.cedarsoftware.util.Converter
import groovy.transform.CompileStatic
import groovy.util.logging.Slf4j
import org.slf4j.Logger
import static com.cedarsoftware.ncube.NCubeAppContext.getNcubeRuntime
@Slf4j
@CompileStatic
class BusinessRule
{
List errors = []
private Object root
Map input
Map output
ApplicationID appId
@SuppressWarnings(value = "unused")
private BusinessRule() {}
BusinessRule(Object root)
{
this.root = root
}
void init(ApplicationID appId, Map input, Map output)
{
input['rule'] = this
input['log'] = log
this.appId = appId
this.input = input
this.output = output
}
Object getRoot()
{
return root
}
Logger getLog()
{
return log
}
/**
* Add an error to a list as rules get executed
* @param category String Group errors by categories if necessary
* @param code String Unique identifier for specific problems
* @param message String English readable message with as much context as possible for fixing the error
*/
void addError(String category, String code, String message)
{
RulesError e = new RulesError(category, code, message)
errors.add(e)
}
/**
* Return a List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy