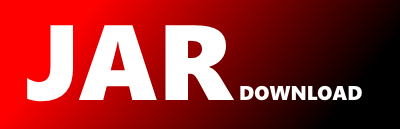
com.cedarsoftware.visualizer.VisualizerCellInfo.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of n-cube Show documentation
Show all versions of n-cube Show documentation
Multi-dimensional Rule Engine
package com.cedarsoftware.visualizer
import com.cedarsoftware.ncube.ApplicationID
import com.cedarsoftware.ncube.NCubeRuntimeClient
import com.cedarsoftware.ncube.exception.CoordinateNotFoundException
import com.cedarsoftware.ncube.exception.InvalidCoordinateException
import com.cedarsoftware.ncube.formatters.HtmlFormatter
import com.google.common.base.Joiner
import groovy.transform.CompileStatic
import static com.cedarsoftware.util.ExceptionUtilities.getDeepestException
import static com.cedarsoftware.visualizer.VisualizerConstants.*
/**
* Provides information to visualize an n-cube cell.
*/
@CompileStatic
class VisualizerCellInfo
{
protected ApplicationID appId
protected String nodeId
protected Map coordinate
protected Object noExecuteCell
protected Object cell
protected Exception exception
protected Joiner.MapJoiner mapJoiner = Joiner.on(", ").withKeyValueSeparator(": ")
protected static NCubeRuntimeClient runtimeClient
protected VisualizerHelper helper = new VisualizerHelper(runtimeClient, appId)
protected VisualizerCellInfo(){}
protected VisualizerCellInfo(NCubeRuntimeClient runtimeClient, ApplicationID appId, String nodeId, Map coordinate)
{
this.runtimeClient = runtimeClient
this.appId = appId
this.coordinate = coordinate
this.nodeId = nodeId
}
protected void getCellValue(VisualizerInfo visInfo, VisualizerRelInfo visRelInfo, Long id, StringBuilder sb)
{
String coordinateString = coordinateString
if (exception)
{
//An exception was caught during the execution of the cell.
sb.append(getExceptionDetails(visInfo, visRelInfo, id, coordinateString))
}
else
{
//The cell has a value or a null value
sb.append(getCellDetails(id))
}
}
private String getCoordinateString()
{
coordinate.each {String scopeKey, Object value ->
if (!value)
{
coordinate[scopeKey] = 'null'
}
}
return mapJoiner.join(coordinate)
}
private String getCellDetails(Long id)
{
String noExecuteValue = HtmlFormatter.getCellValueAsString(noExecuteCell)
String cellString = HtmlFormatter.getCellValueAsString(cell)
StringBuilder sb = new StringBuilder()
String coordinateClassName = "coord_${id}"
String listItemClassName = DETAILS_CLASS_EXECUTED_CELL
String linkClassNames = "${listItemClassName} ${coordinateClassName}"
String preClassNames = "${coordinateClassName} ${DETAILS_CLASS_WORD_WRAP}"
sb.append("""${coordinateString} """)
sb.append("""""")
sb.append("Non-executed value:")
sb.append(DOUBLE_BREAK)
sb.append("${noExecuteValue}")
sb.append(DOUBLE_BREAK)
sb.append("Executed value:")
sb.append(DOUBLE_BREAK)
if (cell && cellString.startsWith(HTTP) || cellString.startsWith(HTTPS) || cellString.startsWith(FILE))
{
sb.append("""${cellString}""")
}
else
{
sb.append("${cellString}")
}
sb.append("
")
return sb.toString()
}
private String getExceptionDetails(VisualizerInfo visInfo, VisualizerRelInfo relInfo, Long id, String coordinateString)
{
StringBuilder sb = new StringBuilder()
StringBuilder mb = new StringBuilder()
String noExecuteValue = HtmlFormatter.getCellValueAsString(noExecuteCell)
Throwable t = getDeepestException(exception)
String listItemClassName
String title
if (t instanceof InvalidCoordinateException)
{
title = 'The cell was executed with a missing or invalid coordinate'
listItemClassName = t.class.simpleName
mb.append("Additional scope is required:${DOUBLE_BREAK}")
mb.append(helper.handleInvalidCoordinateException(t as InvalidCoordinateException, visInfo, relInfo, new LinkedHashSet()).toString())
}
else if (t instanceof CoordinateNotFoundException)
{
title = 'The cell was executed with a missing or invalid coordinate'
listItemClassName = t.class.simpleName
CoordinateNotFoundException exc = t as CoordinateNotFoundException
String scopeKey = exc.axisName
Object value = exc.value ?: 'null'
mb.append("The value ${value} is not valid for ${scopeKey}. A different value must be provided:${DOUBLE_BREAK}")
mb.append(helper.handleCoordinateNotFoundException(t as CoordinateNotFoundException, visInfo, relInfo))
}
else
{
title = 'An error occurred during the execution of the cell'
listItemClassName = DETAILS_CLASS_EXCEPTION
mb.append("An exception was thrown while loading the coordinate.${DOUBLE_BREAK}")
mb.append(helper.handleException(t))
}
String coordinateClassName = "coord_${id}"
String linkClassNames = "${listItemClassName}, ${coordinateClassName}"
String preClassNames = "${coordinateClassName} ${DETAILS_CLASS_WORD_WRAP}"
sb.append("""${coordinateString} """)
sb.append("""""")
sb.append("Non-executed value:")
sb.append(DOUBLE_BREAK)
sb.append("${noExecuteValue}")
sb.append(DOUBLE_BREAK)
sb.append("Exception:")
sb.append(DOUBLE_BREAK)
sb.append("${mb.toString()}>")
sb.append("
")
return sb.toString()
}
Object getCell()
{
return cell
}
void setCell(Object cell)
{
this.cell = cell
}
Exception getException()
{
return exception
}
void setException(Exception exception)
{
this.exception = exception
}
Object getNoExecuteCell()
{
return noExecuteCell
}
void setNoExecuteCell(Object noExecuteCell)
{
this.noExecuteCell = noExecuteCell
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy