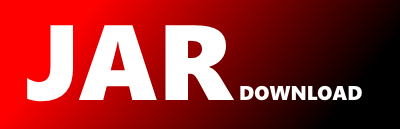
com.celum.dbplugin.installer.SqlInstaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of db-maven-plugin Show documentation
Show all versions of db-maven-plugin Show documentation
DB plugin for Maven that executes SQL scripts
/*****************************************************************************
* Copyright 2012 celum Slovakia s r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*****************************************************************************/
package com.celum.dbplugin.installer;
import com.celum.dbplugin.sqlparser.SqlScriptParser;
import java.io.IOException;
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.*;
/**
* It's main execution class that is doing installation of the DB from scripts.
* The most important method is 'install' that take all SQL scripts from
* {@link SqlScriptsResource} and executes them under {@link com.celum.dbplugin.mojo.DbAccount account}.
*
* @author Zdenko Vrabel ([email protected])
*/
public class SqlInstaller
{
//-------------------------------------------------------------------------------------------------------------------
// members
//-------------------------------------------------------------------------------------------------------------------
/** event handler that reacting on various SqlInstaller's events */
private final SqlInstallerEventHandler eventHandler;
/** this parser split-up the SQL script into statements */
private final SqlScriptParser sqlParser;
//-------------------------------------------------------------------------------------------------------------------
// constructors & methods
//-------------------------------------------------------------------------------------------------------------------
/**
* Constructor
* @param eventHandler
*/
public SqlInstaller(SqlInstallerEventHandler eventHandler)
{
this.eventHandler = eventHandler;
this.sqlParser = new SqlScriptParser();
}
/**
* Constructor
*/
public SqlInstaller()
{
this(new DummyEventHandler());
}
/**
* Executes all SQL scripts from given resources
*
* @param connection
* @param resource
*/
public void install(Connection connection, SqlScriptsResource resource) throws IOException, SQLException
{
Collection scripts = resource.getSortedScripts();
processAllSqlScripts(connection, scripts);
}
private void processAllSqlScripts(Connection con, Collection sqlScripts) throws IOException, SQLException
{
for (SqlScript sqlScript : sqlScripts) {
sqlScript = eventHandler.onProcessScript(sqlScript);
if (sqlScript != null) {
processSql(con, sqlScript);
}
}
}
private void processSql(Connection con, SqlScript script) throws SQLException
{
Statement stm = null;
try {
stm = con.createStatement();
processSqlStatement(stm, script);
} catch (Exception e) {
eventHandler.onError(e);
} finally {
if (stm != null) {
stm.close();
}
}
}
private void processSqlStatement(Statement stm, SqlScript script) throws SQLException
{
Collection sqls = sqlParser.parseScript(script.asText());
for (String sql : sqls) {
sql = eventHandler.onExecuteSql(sql);
stm.execute(sql);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy