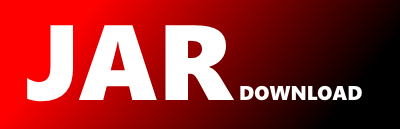
com.celum.dbplugin.mojo.AbstractDbMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of db-maven-plugin Show documentation
Show all versions of db-maven-plugin Show documentation
DB plugin for Maven that executes SQL scripts
/*****************************************************************************
* Copyright 2012 celum Slovakia s r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*****************************************************************************/
package com.celum.dbplugin.mojo;
import com.celum.dbplugin.installer.SqlInstaller;
import com.celum.dbplugin.installer.SqlInstallerEventHandler;
import com.celum.dbplugin.installer.SqlScript;
import com.celum.dbplugin.installer.SqlScriptsResource;
import com.celum.dbplugin.resource.DbVersionFilter;
import com.celum.dbplugin.resource.SqlDirResource;
import com.celum.dbplugin.resource.VelocityDecoratorResource;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import java.io.File;
import java.util.Map;
/**
* @author Zdenko Vrabel ([email protected])
*/
public abstract class AbstractDbMojo extends AbstractMojo implements SqlInstallerEventHandler
{
//-------------------------------------------------------------------------------------------------------------------
// members & mojo parameters
//-------------------------------------------------------------------------------------------------------------------
/**
* @parameter
*/
private Map templateContext;
/**
* @parameter expression="${versionSql}"
*/
private String versionSql;
/**
* @parameter
*/
private String stepPattern;
/**
* @parameter
*/
protected DbAccount adminAccount;
/**
* @parameter
*/
protected DbAccount appAccount;
/**
* @parameter default-value="${basedir}/src/main/sql/create"
*/
protected File createScripts;
/**
* @parameter default-value="${basedir}/src/main/sql/scheme"
*/
protected File schemeScripts;
/**
* @parameter default-value="${basedir}/src/main/sql/patch"
*/
protected File patchScripts;
/**
* @parameter default-value="${basedir}/src/main/sql/drop"
*/
protected File dropScripts;
//-------------------------------------------------------------------------------------------------------------------
// methods
//-------------------------------------------------------------------------------------------------------------------
@Override
public SqlScript onProcessScript(SqlScript sqlScript)
{
getLog().info("process script:" + sqlScript.getId());
return sqlScript;
}
@Override
public String onExecuteSql(String sql)
{
getLog().info("sql:" + sql.trim());
return sql;
}
@Override
public void onError(Throwable e)
{
getLog().error(e);
}
protected void install(DbAccount account, File scriptsDir) throws MojoExecutionException, MojoFailureException
{
try {
SqlScriptsResource res = new SqlDirResource(scriptsDir);
if (templateContext != null) {
res = new VelocityDecoratorResource(res, templateContext);
}
SqlInstaller installer = new SqlInstaller(this);
installer.install(account.getConnection(), res);
} catch (Exception e) {
getLog().error(e);
throw new MojoFailureException(e.getMessage());
} finally {
account.closeConnectionQuietly();
}
}
protected void upgrade(DbAccount account, File scriptsDir) throws MojoExecutionException, MojoFailureException
{
try {
SqlScriptsResource res = new SqlDirResource(scriptsDir);
if (versionSql != null && !versionSql.isEmpty()) {
res = new DbVersionFilter(res, account.getConnection(), versionSql, stepPattern);
}
if (templateContext != null) {
res = new VelocityDecoratorResource(res, templateContext);
}
SqlInstaller installer = new SqlInstaller(this);
installer.install(account.getConnection(), res);
} catch (Exception e) {
getLog().error(e);
throw new MojoFailureException(e.getMessage());
} finally {
account.closeConnectionQuietly();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy