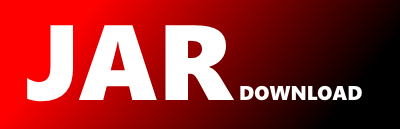
com.celum.dbplugin.sqlparser.SqlGrammarLexer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of db-maven-plugin Show documentation
Show all versions of db-maven-plugin Show documentation
DB plugin for Maven that executes SQL scripts
// $ANTLR 3.3 Nov 30, 2010 12:46:29 com/celum/dbplugin/sqlparser/SqlGrammar.g 2012-11-20 09:51:37
package com.celum.dbplugin.sqlparser;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
public class SqlGrammarLexer extends Lexer {
public static final int EOF=-1;
public static final int Statement=4;
public static final int StringVal=5;
public static final int String2Val=6;
public static final int SimpleVal=7;
// delegates
// delegators
public SqlGrammarLexer() {;}
public SqlGrammarLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public SqlGrammarLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
public String getGrammarFileName() { return "com/celum/dbplugin/sqlparser/SqlGrammar.g"; }
// $ANTLR start "Statement"
public final void mStatement() throws RecognitionException {
try {
int _type = Statement;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/celum/dbplugin/sqlparser/SqlGrammar.g:22:2: ( ( StringVal | String2Val | SimpleVal )+ ';' )
// com/celum/dbplugin/sqlparser/SqlGrammar.g:22:4: ( StringVal | String2Val | SimpleVal )+ ';'
{
// com/celum/dbplugin/sqlparser/SqlGrammar.g:22:4: ( StringVal | String2Val | SimpleVal )+
int cnt1=0;
loop1:
do {
int alt1=4;
int LA1_0 = input.LA(1);
if ( (LA1_0=='\"') ) {
alt1=1;
}
else if ( (LA1_0=='\'') ) {
alt1=2;
}
else if ( ((LA1_0>='\u0000' && LA1_0<='!')||(LA1_0>='#' && LA1_0<='&')||(LA1_0>='(' && LA1_0<=':')||(LA1_0>='<' && LA1_0<='\uFFFF')) ) {
alt1=3;
}
switch (alt1) {
case 1 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:22:5: StringVal
{
mStringVal();
}
break;
case 2 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:22:17: String2Val
{
mString2Val();
}
break;
case 3 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:22:30: SimpleVal
{
mSimpleVal();
}
break;
default :
if ( cnt1 >= 1 ) break loop1;
EarlyExitException eee =
new EarlyExitException(1, input);
throw eee;
}
cnt1++;
} while (true);
match(';');
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "Statement"
// $ANTLR start "StringVal"
public final void mStringVal() throws RecognitionException {
try {
int _type = StringVal;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/celum/dbplugin/sqlparser/SqlGrammar.g:26:2: ( ( '\"' (~ '\"' )* '\"' ) )
// com/celum/dbplugin/sqlparser/SqlGrammar.g:26:4: ( '\"' (~ '\"' )* '\"' )
{
// com/celum/dbplugin/sqlparser/SqlGrammar.g:26:4: ( '\"' (~ '\"' )* '\"' )
// com/celum/dbplugin/sqlparser/SqlGrammar.g:26:5: '\"' (~ '\"' )* '\"'
{
match('\"');
// com/celum/dbplugin/sqlparser/SqlGrammar.g:26:9: (~ '\"' )*
loop2:
do {
int alt2=2;
int LA2_0 = input.LA(1);
if ( ((LA2_0>='\u0000' && LA2_0<='!')||(LA2_0>='#' && LA2_0<='\uFFFF')) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:26:10: ~ '\"'
{
if ( (input.LA(1)>='\u0000' && input.LA(1)<='!')||(input.LA(1)>='#' && input.LA(1)<='\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
default :
break loop2;
}
} while (true);
match('\"');
}
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "StringVal"
// $ANTLR start "String2Val"
public final void mString2Val() throws RecognitionException {
try {
int _type = String2Val;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/celum/dbplugin/sqlparser/SqlGrammar.g:30:2: ( ( '\\'' (~ '\\'' )* '\\'' ) )
// com/celum/dbplugin/sqlparser/SqlGrammar.g:30:4: ( '\\'' (~ '\\'' )* '\\'' )
{
// com/celum/dbplugin/sqlparser/SqlGrammar.g:30:4: ( '\\'' (~ '\\'' )* '\\'' )
// com/celum/dbplugin/sqlparser/SqlGrammar.g:30:5: '\\'' (~ '\\'' )* '\\''
{
match('\'');
// com/celum/dbplugin/sqlparser/SqlGrammar.g:30:10: (~ '\\'' )*
loop3:
do {
int alt3=2;
int LA3_0 = input.LA(1);
if ( ((LA3_0>='\u0000' && LA3_0<='&')||(LA3_0>='(' && LA3_0<='\uFFFF')) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:30:11: ~ '\\''
{
if ( (input.LA(1)>='\u0000' && input.LA(1)<='&')||(input.LA(1)>='(' && input.LA(1)<='\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
default :
break loop3;
}
} while (true);
match('\'');
}
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "String2Val"
// $ANTLR start "SimpleVal"
public final void mSimpleVal() throws RecognitionException {
try {
int _type = SimpleVal;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/celum/dbplugin/sqlparser/SqlGrammar.g:34:2: ( (~ ( '\\'' | '\"' | ';' ) )+ )
// com/celum/dbplugin/sqlparser/SqlGrammar.g:34:4: (~ ( '\\'' | '\"' | ';' ) )+
{
// com/celum/dbplugin/sqlparser/SqlGrammar.g:34:4: (~ ( '\\'' | '\"' | ';' ) )+
int cnt4=0;
loop4:
do {
int alt4=2;
int LA4_0 = input.LA(1);
if ( ((LA4_0>='\u0000' && LA4_0<='!')||(LA4_0>='#' && LA4_0<='&')||(LA4_0>='(' && LA4_0<=':')||(LA4_0>='<' && LA4_0<='\uFFFF')) ) {
alt4=1;
}
switch (alt4) {
case 1 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:34:4: ~ ( '\\'' | '\"' | ';' )
{
if ( (input.LA(1)>='\u0000' && input.LA(1)<='!')||(input.LA(1)>='#' && input.LA(1)<='&')||(input.LA(1)>='(' && input.LA(1)<=':')||(input.LA(1)>='<' && input.LA(1)<='\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;}
}
break;
default :
if ( cnt4 >= 1 ) break loop4;
EarlyExitException eee =
new EarlyExitException(4, input);
throw eee;
}
cnt4++;
} while (true);
}
state.type = _type;
state.channel = _channel;
}
finally {
}
}
// $ANTLR end "SimpleVal"
public void mTokens() throws RecognitionException {
// com/celum/dbplugin/sqlparser/SqlGrammar.g:1:8: ( Statement | StringVal | String2Val | SimpleVal )
int alt5=4;
alt5 = dfa5.predict(input);
switch (alt5) {
case 1 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:1:10: Statement
{
mStatement();
}
break;
case 2 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:1:20: StringVal
{
mStringVal();
}
break;
case 3 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:1:30: String2Val
{
mString2Val();
}
break;
case 4 :
// com/celum/dbplugin/sqlparser/SqlGrammar.g:1:41: SimpleVal
{
mSimpleVal();
}
break;
}
}
protected DFA5 dfa5 = new DFA5(this);
static final String DFA5_eotS =
"\3\uffff\1\10\1\uffff\1\12\1\uffff\1\13\4\uffff";
static final String DFA5_eofS =
"\14\uffff";
static final String DFA5_minS =
"\10\0\4\uffff";
static final String DFA5_maxS =
"\10\uffff\4\uffff";
static final String DFA5_acceptS =
"\10\uffff\1\4\1\1\1\2\1\3";
static final String DFA5_specialS =
"\1\0\1\2\1\1\1\3\1\7\1\6\1\4\1\5\4\uffff}>";
static final String[] DFA5_transitionS = {
"\42\3\1\1\4\3\1\2\23\3\1\uffff\uffc4\3",
"\42\4\1\5\uffdd\4",
"\47\6\1\7\uffd8\6",
"\42\3\1\11\4\3\1\11\23\3\1\11\uffc4\3",
"\42\4\1\5\uffdd\4",
"\0\11",
"\47\6\1\7\uffd8\6",
"\0\11",
"",
"",
"",
""
};
static final short[] DFA5_eot = DFA.unpackEncodedString(DFA5_eotS);
static final short[] DFA5_eof = DFA.unpackEncodedString(DFA5_eofS);
static final char[] DFA5_min = DFA.unpackEncodedStringToUnsignedChars(DFA5_minS);
static final char[] DFA5_max = DFA.unpackEncodedStringToUnsignedChars(DFA5_maxS);
static final short[] DFA5_accept = DFA.unpackEncodedString(DFA5_acceptS);
static final short[] DFA5_special = DFA.unpackEncodedString(DFA5_specialS);
static final short[][] DFA5_transition;
static {
int numStates = DFA5_transitionS.length;
DFA5_transition = new short[numStates][];
for (int i=0; i='\u0000' && LA5_0<='!')||(LA5_0>='#' && LA5_0<='&')||(LA5_0>='(' && LA5_0<=':')||(LA5_0>='<' && LA5_0<='\uFFFF')) ) {s = 3;}
if ( s>=0 ) return s;
break;
case 1 :
int LA5_2 = input.LA(1);
s = -1;
if ( ((LA5_2>='\u0000' && LA5_2<='&')||(LA5_2>='(' && LA5_2<='\uFFFF')) ) {s = 6;}
else if ( (LA5_2=='\'') ) {s = 7;}
if ( s>=0 ) return s;
break;
case 2 :
int LA5_1 = input.LA(1);
s = -1;
if ( ((LA5_1>='\u0000' && LA5_1<='!')||(LA5_1>='#' && LA5_1<='\uFFFF')) ) {s = 4;}
else if ( (LA5_1=='\"') ) {s = 5;}
if ( s>=0 ) return s;
break;
case 3 :
int LA5_3 = input.LA(1);
s = -1;
if ( (LA5_3=='\"'||LA5_3=='\''||LA5_3==';') ) {s = 9;}
else if ( ((LA5_3>='\u0000' && LA5_3<='!')||(LA5_3>='#' && LA5_3<='&')||(LA5_3>='(' && LA5_3<=':')||(LA5_3>='<' && LA5_3<='\uFFFF')) ) {s = 3;}
else s = 8;
if ( s>=0 ) return s;
break;
case 4 :
int LA5_6 = input.LA(1);
s = -1;
if ( (LA5_6=='\'') ) {s = 7;}
else if ( ((LA5_6>='\u0000' && LA5_6<='&')||(LA5_6>='(' && LA5_6<='\uFFFF')) ) {s = 6;}
if ( s>=0 ) return s;
break;
case 5 :
int LA5_7 = input.LA(1);
s = -1;
if ( ((LA5_7>='\u0000' && LA5_7<='\uFFFF')) ) {s = 9;}
else s = 11;
if ( s>=0 ) return s;
break;
case 6 :
int LA5_5 = input.LA(1);
s = -1;
if ( ((LA5_5>='\u0000' && LA5_5<='\uFFFF')) ) {s = 9;}
else s = 10;
if ( s>=0 ) return s;
break;
case 7 :
int LA5_4 = input.LA(1);
s = -1;
if ( (LA5_4=='\"') ) {s = 5;}
else if ( ((LA5_4>='\u0000' && LA5_4<='!')||(LA5_4>='#' && LA5_4<='\uFFFF')) ) {s = 4;}
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 5, _s, input);
error(nvae);
throw nvae;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy