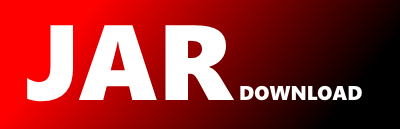
com.celum.dbplugin.sqlparser.SqlScriptParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of db-maven-plugin Show documentation
Show all versions of db-maven-plugin Show documentation
DB plugin for Maven that executes SQL scripts
/*****************************************************************************
* Copyright 2012 celum Slovakia s r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*****************************************************************************/
package com.celum.dbplugin.sqlparser;
import org.antlr.runtime.*;
import java.io.*;
import java.util.*;
/**
* Class parse the whole SQL script into SQL statements. This is
* basically facade that is hiding ANTLR grammar for SQL script.
*
* @author Zdenko Vrabel ([email protected])
*/
public class SqlScriptParser
{
/**
* Method parse SQL script and returns collection of SQL Statements
* @param script
* @return
*/
public Collection parseScript(String script)
{
try {
return parseScript(new ANTLRStringStream(script));
} catch (Exception e) {
throw new IllegalStateException(e);
}
}
/**
* Method parse SQL script and returns collection of SQL Statements
* @param script
* @return
* @throws IOException
*/
public Collection parseScript(InputStream script) throws Exception
{
return parseScript(new ANTLRInputStream(script));
}
/**
* Method parse SQL script and returns collection of SQL Statements
*
* @param scriptStream
* @return
*/
private Collection parseScript(CharStream scriptStream) throws IOException, RecognitionException
{
//parse script
SqlGrammarLexer lexer = new SqlGrammarLexer(scriptStream);
CommonTokenStream tokens = new CommonTokenStream(lexer);
SqlGrammarParser parser = new SqlGrammarParser(tokens);
parser.sqlscript();
//process tokens
List statements = new LinkedList();
for(Object o : tokens.getTokens()) {
CommonToken token = (CommonToken)o;
String statement = token.getText();
statement = removeMess(statement);
if (!statement.isEmpty()) {
statements.add(statement);
}
}
return statements;
}
/**
* Method remove mess from SQL statement like comments, termination character
* ';' etc.
*
* @param statement
* @return clean statement
*/
private String removeMess(String statement)
{
StringBuffer sb = new StringBuffer();
Scanner scanner = new Scanner(statement);
while (scanner.hasNextLine())
{
String line = scanner.nextLine();
if (line.startsWith("--")) {
continue;
}
if (line.isEmpty()) {
continue;
}
if (line.startsWith("")) {
return "";
}
line = line.trim();
if (line.endsWith(";")) {
line = line.substring(0, line.length() - 1);
}
sb.append(line + "\n");
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy