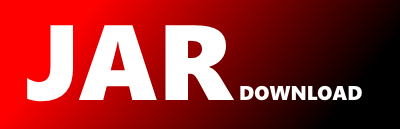
com.celum.dbtool.step.RegularVersion Maven / Gradle / Ivy
/*****************************************************************************
* Copyright 2012 celum Slovakia s r.o.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*****************************************************************************/
package com.celum.dbtool.step;
/**
* Representing regular software version in 4-number
* format major[.minor[.build]].step
*
* @author Zdenko Vrabel ([email protected])
*/
public class RegularVersion extends Version
{
private final long major;
private final long minor;
private final long build;
private final long step;
/**
* Constructor
*/
public RegularVersion(long major, long minor, long build, long step)
{
this.major = major;
this.minor = minor;
this.build = build;
this.step = step;
}
/**
* Constructor
*/
public RegularVersion(long major, long minor, long step)
{
this(major, minor, -1, step);
}
/**
* Constructor
*/
public RegularVersion(long major, long step)
{
this(major, -1, step);
}
/**
* Constructor
*/
public RegularVersion(long step)
{
this(0, -1, step);
}
/**
* returns major number
*/
public long getMajor()
{
return major;
}
/**
* returns minor number
*/
public long getMinor()
{
return minor;
}
/**
* returns build number
*/
public long getBuild()
{
return build;
}
/**
* returns DB migration step
*/
public long getStep()
{
return step;
}
/**
* {@inheritDoc}
* @return
*/
@Override
public String toString()
{
String version = Long.toString(major);
if (minor >= 0) {
version += "." + minor;
}
if (build >= 0) {
version += "." + build;
}
version += "." + step;
return version;
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
int result = (int) (major ^ (major >>> 32));
result = 31 * result + (int) (minor ^ (minor >>> 32));
result = 31 * result + (int) (build ^ (build >>> 32));
result = 31 * result + (int) (step ^ (step >>> 32));
return result;
}
/**
* {@inheritDoc}
*/
@Override
public int compareTo(Version o)
{
if (!(o instanceof RegularVersion)) {
throw new VersionException("cannot compare RegularVersion with " + o.getClass().getName() );
}
RegularVersion version = (RegularVersion)o;
//compare major
if (major < version.major) {
return -1;
} else if (major > version.major) {
return 1;
}
//compare minor
if (minor < version.minor) {
return -1;
} else if (minor > version.minor) {
return 1;
}
//compare build
if (build < version.build) {
return -1;
} else if (build > version.build) {
return 1;
}
//compare build
if (step < version.step) {
return -1;
} else if (step > version.step) {
return 1;
}
return 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy