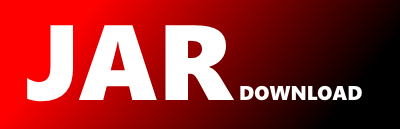
com.centit.support.database.jsonmaptable.MySqlJsonObjectDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of centit-database Show documentation
Show all versions of centit-database Show documentation
数据库操作通用方法和函数,从以前的util包中分离出来,并且整合了部分sys-module中的函数
package com.centit.support.database.jsonmaptable;
import java.io.IOException;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.tuple.Pair;
import com.alibaba.fastjson.JSONArray;
import com.centit.support.database.DatabaseAccess;
import com.centit.support.database.QueryUtils;
import com.centit.support.database.metadata.TableInfo;
public class MySqlJsonObjectDao extends GeneralJsonObjectDao {
public MySqlJsonObjectDao(){
}
public MySqlJsonObjectDao(Connection conn) {
super(conn);
}
public MySqlJsonObjectDao(TableInfo tableInfo) {
super(tableInfo);
}
public MySqlJsonObjectDao(Connection conn,TableInfo tableInfo) {
super(conn,tableInfo);
}
@Override
public JSONArray listObjectsByProperties(final Map properties,
final int startPos,final int maxSize)
throws SQLException, IOException {
TableInfo tableInfo = this.getTableInfo();
Pair q = buildFieldSql(tableInfo,null);
String filter = buildFilterSql(tableInfo,null,properties.keySet());
String sql = "select " + q.getLeft() +" from " +tableInfo.getTableName();
if(StringUtils.isNotBlank(filter))
sql = sql + " where " + filter;
return DatabaseAccess.findObjectsByNamedSqlAsJSON(
getConnect(),
QueryUtils.buildMySqlLimitQuerySQL(
sql,
startPos, maxSize, false),
properties,
q.getRight());
}
@Override
public Long getSequenceNextValue(final String sequenceName) throws SQLException, IOException {
return getSimulateSequenceNextValue(sequenceName);
/*Object object = DatabaseAccess.getScalarObjectQuery(
getConnect(),
"SELECT sequence_nextval ('"+ sequenceName+"')");
return NumberBaseOpt.castObjectToLong(object);*/
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy