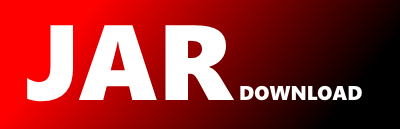
com.centit.search.document.FileDocument Maven / Gradle / Ivy
package com.centit.search.document;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.centit.search.annotation.ESType;
import com.centit.support.json.JSONOpt;
import java.io.Serializable;
import java.util.Date;
/**
* Created by codefan on 17-6-1.
*/
@ESType(type="file")
public class FileDocument implements ESDocument, Serializable {
public static final String ES_DOCUMENT_TYPE = "file";
private static final long serialVersionUID = 1L;
/**
* 所属系统
*/
@ESType(type="text")
private String osId;
/**
* 所属业务
*/
@ESType(type="text")
private String optId;
/**
* 关联的业务对象主键 键值对形式
*/
@ESType(type="text")
private String optTag;
/**
* 关联的方法 可以为空
*/
@ESType(type="text")
private String optMethod;
/**
* 文档反向关联url
*/
@ESType(type="text")
private String optUrl;
/**
* 所属人员 可以为空
*/
@ESType(type="text")
private String userCode;
/**
* 所属机构 可以为空
*/
@ESType(type="text")
private String unitCode;
/**
* 文档名称
*/
@ESType(type="text",index = "analyzed", analyzer = "ik_smart",query = true)
private String fileName;
/**
* 文档的摘要
*/
@ESType(type="text",index = "analyzed", query = true, revert = false, highlight = true,analyzer = "ik_smart")
private String fileSummary;
/**
* 文档的ID
*/
@ESType(type="text")
private String fileId;
/**
* 文档的Md5
*/
@ESType(type="text")
private String fileMD5;
/**
* 文档的内容,用于索引
*/
@ESType(type="text",index = "analyzed", query = true, revert = false, highlight = true,analyzer = "ik_smart")
private String content;
/**
* 文档创建时间
*/
@ESType(type="date")
private Date createTime;
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof FileDocument)) return false;
FileDocument that = (FileDocument) o;
if (!getOsId().equals(that.getOsId())) return false;
if (!getFileId().equals(that.getFileId())) return false;
if (!getFileMD5().equals(that.getFileMD5())) return false;
return true;
}
@Override
public int hashCode() {
int result = getOsId().hashCode();
result = 31 * result + getFileId().hashCode();
result = 31 * result + getFileMD5().hashCode();
return result;
}
@Override
public String toString(){
return toJsonString();
}
public String toJsonString(){
return JSON.toJSONString(this);
}
public String getOsId() {
return osId;
}
public void setOsId(String osId) {
this.osId = osId;
}
public String getOptId() {
return optId;
}
public void setOptId(String optId) {
this.optId = optId;
}
public String getOptMethod() {
return optMethod;
}
public void setOptMethod(String optMethod) {
this.optMethod = optMethod;
}
public String getOptTag() {
return optTag;
}
public void setOptTag(String optTag) {
this.optTag = optTag;
}
public String getOptUrl() {
return optUrl;
}
public void setOptUrl(String optUrl) {
this.optUrl = optUrl;
}
public String getUserCode() {
return userCode;
}
public void setUserCode(String userCode) {
this.userCode = userCode;
}
public String getUnitCode() {
return unitCode;
}
public void setUnitCode(String unitCode) {
this.unitCode = unitCode;
}
public String getFileName() {
return fileName;
}
public void setFileName(String fileName) {
this.fileName = fileName;
}
public String getFileSummary() {
return fileSummary;
}
public void setFileSummary(String fileSummary) {
this.fileSummary = fileSummary;
}
public String getFileId() {
return fileId;
}
public void setFileId(String fileId) {
this.fileId = fileId;
}
public String getFileMD5() {
return fileMD5;
}
public void setFileMD5(String fileMD5) {
this.fileMD5 = fileMD5;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public Date getCreateTime() {
return createTime;
}
public void setCreateTime(Date createTime) {
this.createTime = createTime;
}
@Override
//@JSONField(serialize=false,deserialize=false)
public String obtainDocumentId() {
return fileId;
}
@Override
//@JSONField(serialize=false,deserialize=false)
public String obtainDocumentType() {
return ES_DOCUMENT_TYPE;
}
@Override
public JSONObject toJSONObject() {
return (JSONObject)JSON.toJSON(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy