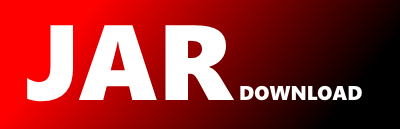
com.centit.search.service.Impl.ESIndexer Maven / Gradle / Ivy
package com.centit.search.service.Impl;
import com.centit.search.document.DocumentUtils;
import com.centit.search.document.ESDocument;
import com.centit.search.service.ESServerConfig;
import com.centit.search.service.Indexer;
import org.apache.commons.pool2.impl.GenericObjectPool;
import org.apache.commons.pool2.impl.GenericObjectPoolConfig;
import org.elasticsearch.action.admin.indices.exists.indices.IndicesExistsRequest;
import org.elasticsearch.action.admin.indices.exists.indices.IndicesExistsResponse;
import org.elasticsearch.action.admin.indices.mapping.put.PutMappingRequest;
import org.elasticsearch.action.delete.DeleteResponse;
import org.elasticsearch.action.get.GetResponse;
import org.elasticsearch.action.index.IndexResponse;
import org.elasticsearch.action.update.UpdateResponse;
import org.elasticsearch.client.Requests;
import org.elasticsearch.client.transport.TransportClient;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Created by codefan on 17-6-12.
*/
public class ESIndexer implements Indexer{
private static Logger logger = LoggerFactory.getLogger(ESIndexer.class);
private ESServerConfig config;
private GenericObjectPool clientPool;
public ESIndexer(ESServerConfig config){
this.config = config;
GenericObjectPoolConfig poolConfig = new GenericObjectPoolConfig();
clientPool = new GenericObjectPool<>(new PooledTransportClientTactory(config),
poolConfig);
}
public boolean createIndexIfNotExist() {
TransportClient client = null;
try {
client = clientPool.borrowObject();
IndicesExistsResponse indicesExistsResponse = client.admin().indices()
.exists(new IndicesExistsRequest(config.getIndexName()))
.actionGet();
if (!indicesExistsResponse.isExists()) {
client.admin().indices().prepareCreate(config.getIndexName()).execute().actionGet();
return true;
}
return false;
} catch (Exception e) {
logger.error(e.getMessage(), e);
return false;
}finally {
if(client!=null)
clientPool.returnObject(client);
}
}
// 定义索引的映射类型
public void initMappingType(Class> ... objTypes) {
//assert(StringUtils.equals(document.getOsId(),config.getOsId()));
//判断索引是否存在,不存在则新建
TransportClient client = null;
try {
client = clientPool.borrowObject();
if(objTypes!=null){
for(Class> objType : objTypes) {
String documentType = DocumentUtils.obtainDocumentType(objType);
if (documentType != null) {
PutMappingRequest putMappingRequest
= Requests.putMappingRequest((config.getIndexName()))
.type(documentType).source(DocumentUtils.obtainDocumentMapping(objType));
client.admin().indices().putMapping(putMappingRequest).actionGet();
}
}
}
//return client;
} catch (Exception e) {
logger.error(e.getMessage(), e);
}finally {
if(client!=null)
clientPool.returnObject(client);
}
}
/**
* 新建 文档
* @param document 文档
* @return 返回文档的 文档ID
*/
@Override
public String saveNewDocument(ESDocument document) {
TransportClient client = null;
try {
client = clientPool.borrowObject();
String type = document.obtainDocumentType();
String docId = document.obtainDocumentId();
IndexResponse indexResponse = client.prepareIndex(
config.getIndexName(), type, docId).setSource(
document.toJSONObject()).execute().actionGet();
return indexResponse.getId();
}
catch (Exception e) {
logger.error(e.getMessage(), e);
return null;
}finally {
if(client!=null)
clientPool.returnObject(client);
}
}
/**
* 根据文档ID 删除文档
*
* @param document 文档
* @return 返回布尔类型
*/
@Override
public boolean deleteDocument(ESDocument document) {
TransportClient client = null;
try {
client = clientPool.borrowObject();
String type = document.obtainDocumentType();
String docId = document.obtainDocumentId();
DeleteResponse response = client.prepareDelete( config.getIndexName() , type, docId).get();
return response.status().getStatus() == 200;
}
catch (Exception e) {
logger.error(e.getMessage(), e);
return false;
}finally {
if(client!=null)
clientPool.returnObject(client);
}
}
/**
* 根据文件类型和ID 删除文档
* @param docType String 文档类型
* @param docId String 文档id
* @return 删除返回的布尔类型
*/
@Override
public boolean deleteDocument(String docType,String docId) {
TransportClient client = null;
try {
client = clientPool.borrowObject();
DeleteResponse response = client.prepareDelete(
config.getIndexName() , docType, docId).execute().actionGet();
return response.status().getStatus() == 200;
}
catch (Exception e) {
logger.error(e.getMessage(), e);
return false;
}finally {
if(client!=null)
clientPool.returnObject(client);
}
}
/**
* 更新文档
*
* @param document 要跟新的文档对象
* @return 更新后返回int值
*/
@Override
public int updateDocument(ESDocument document) {
String docId = document.obtainDocumentId();
return updateDocument(docId, document);
}
/**
* 更新文档
*
* @param docId 文档id
* @param document 要更新的文档对象
* @return 更新后返回的int值
*/
@Override
public int updateDocument(String docId, ESDocument document) {
TransportClient client = null;
try {
client = clientPool.borrowObject();
String type = document.obtainDocumentType();
UpdateResponse response = client.prepareUpdate(config.getIndexName(), type, docId)
.setDoc(document.toJSONObject()).get();
int ret = response.status().getStatus();
return (ret == 200)?1:0;
}
catch (Exception e) {
logger.error(e.getMessage(), e);
return 0;
}finally {
if(client!=null)
clientPool.returnObject(client);
}
}
/**
* 合并文档
*
* @param document ESDocument
* @return 是否成功
*/
@Override
public String mergeDocument(ESDocument document) {
TransportClient client = null;
try {
client = clientPool.borrowObject();
String type = document.obtainDocumentType();
String docId = document.obtainDocumentId();
GetResponse response = client.prepareGet(config.getIndexName(),
type,docId).get();
if(response.isExists()){
UpdateResponse updateResponse = client.prepareUpdate(config.getIndexName(), type, docId)
.setDoc(document.toJSONObject()).get();
int ret = updateResponse.status().getStatus();
return (ret == 200)? updateResponse.getId() : null;
}else{
IndexResponse indexResponse = client.prepareIndex(
config.getIndexName(), type, docId).setSource(
document.toJSONObject()).execute().actionGet();
return indexResponse.getId();
}
}
catch (Exception e) {
logger.error(e.getMessage(), e);
return null;
}finally {
if(client!=null)
clientPool.returnObject(client);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy