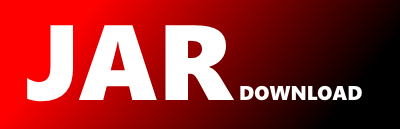
com.centit.support.algorithm.ByteBaseOpt Maven / Gradle / Ivy
package com.centit.support.algorithm;
import java.lang.reflect.Array;
import java.util.Date;
@SuppressWarnings("unused")
public abstract class ByteBaseOpt {
private ByteBaseOpt() {
throw new IllegalAccessError("Utility class");
}
public static byte[] castObjectToBytes(Object obj) {
if (obj instanceof byte[]) {
return (byte[]) obj;
}
if (obj.getClass().isArray()) {
int len = Array.getLength(obj);
if (len == 0) {
return null;
}
Object firstObj = Array.get(obj, 0);
if (firstObj instanceof Byte) {
byte[] bytes = new byte[len];
for (int i = 0; i < len; i++) {
Object bObj = Array.get(obj, i);
bytes[i] = (Byte) bObj;
}
return bytes;
}
if (firstObj instanceof Character) {
byte[] bytes = new byte[len];
for (int i = 0; i < len; i++) {
Object bObj = Array.get(obj, i);
bytes[i] = (byte) ((Character) bObj).charValue();
}
return bytes;
}
return StringBaseOpt.objectToString(obj).getBytes();
}
if (obj instanceof Long) {
byte[] buf = new byte[8];
writeInt64(buf, (Long) obj, 0);
return buf;
}
if (obj instanceof Integer) {
byte[] buf = new byte[4];
writeInt32(buf, (Integer) obj, 0);
return buf;
}
if (obj instanceof Date) {
byte[] buf = new byte[8];
writeInt64(buf, ((Date) obj).getTime(), 0);
return buf;
}
if (obj instanceof Float) {
byte[] buf = new byte[4];
writeFloat(buf, (Float) obj, 0);
return buf;
}
if (obj instanceof Double) {
byte[] buf = new byte[8];
writeDouble(buf, (Double) obj, 0);
return buf;
}
return StringBaseOpt.objectToString(obj).getBytes();
}
public static int writeInt64(byte[] buf, long data, int offset) {
for (int i = 0; i < 8; i++) {
buf[offset + 7 - i] = (byte) (data & 0xff);
data = data >> 8;
}
return offset + 8;
}
public static int writeLong(byte[] buf, long data, int offset) {
return writeInt64(buf, data, offset);
}
public static int writeInt32(byte[] buf, int data, int offset) {
for (int i = 0; i < 4; i++) {
buf[offset + 3 - i] = (byte) (data & 0xff);
data = data >> 8;
}
return offset + 4;
}
public static int writeUInt32(byte[] buf, long data, int offset) {
for (int i = 0; i < 4; i++) {
buf[offset + 3 - i] = (byte) (data & 0xff);
data = data >> 8;
}
return offset + 4;
}
public static int writeInt(byte[] buf, int data, int offset) {
return writeInt32(buf, data, offset);
}
public static int writeInt16(byte[] buf, short data, int offset) {
for (int i = 0; i < 2; i++) {
buf[offset + 1 - i] = Integer.valueOf(data & 0xff).byteValue();
data = (short) (data >> 8);
}
return offset + 2;
}
public static int writeUInt16(byte[] buf, int data, int offset) {
for (int i = 0; i < 2; i++) {
buf[offset + 1 - i] = (byte) (data & 0xff);
data = (short) (data >> 8);
}
return offset + 2;
}
public static int writeShort(byte[] buf, short data, int offset) {
return writeInt16(buf, data, offset);
}
public static int writeFloat(byte[] buf, float data, int offset) {
int intDate = Float.floatToIntBits(data);
return writeInt32(buf, intDate, offset);
}
public static int writeDouble(byte[] buf, double data, int offset) {
long longDate = Double.doubleToLongBits(data);
return writeInt64(buf, longDate, offset);
}
public static int writeStringAsBytes(byte[] buf, String data, int offset) {
byte[] strBytes = data.getBytes();
System.arraycopy(strBytes, 0, buf, offset, strBytes.length);
/*for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy