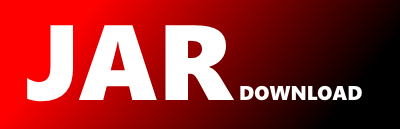
com.centurylink.mdw.export.ExportHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mdw-common Show documentation
Show all versions of mdw-common Show documentation
MDW is a microservices based workflow framework
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy