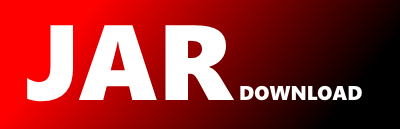
com.centurylink.mdw.html.HtmlExportHelper Maven / Gradle / Ivy
/*
* Copyright (C) 2018 CenturyLink, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.centurylink.mdw.html;
import com.centurylink.mdw.constant.WorkAttributeConstant;
import com.centurylink.mdw.image.ImageExportHelper;
import com.centurylink.mdw.model.attribute.Attribute;
import com.centurylink.mdw.model.project.Project;
import com.centurylink.mdw.model.variable.Variable;
import com.centurylink.mdw.model.workflow.Activity;
import com.centurylink.mdw.model.workflow.ActivityNodeSequencer;
import com.centurylink.mdw.model.workflow.Process;
import com.vladsch.flexmark.ast.Node;
import com.vladsch.flexmark.html.HtmlRenderer;
import com.vladsch.flexmark.parser.Parser;
import javax.imageio.ImageIO;
import java.io.File;
import java.io.IOException;
import java.util.*;
public class HtmlExportHelper {
public static final String BR = "
";
public static final String HTMLTAG = "";
public static final String ACTIVITY = "Activity ";
private Set excludedAttributes;
private Map> tabularAttributes; // name, headers
private Map textboxAttributes;
private Map excludedAttributesForSpecificValues;
private Project project;
public HtmlExportHelper(Project project) {
this.project = project;
excludedAttributes = new HashSet<>();
excludedAttributes.add(WorkAttributeConstant.LOGICAL_ID);
excludedAttributes.add(WorkAttributeConstant.REFERENCE_ID);
excludedAttributes.add(WorkAttributeConstant.WORK_DISPLAY_INFO);
excludedAttributes.add(WorkAttributeConstant.REFERENCES);
excludedAttributes.add(WorkAttributeConstant.DOCUMENTATION);
excludedAttributes.add(WorkAttributeConstant.SIMULATION_STUB_MODE);
excludedAttributes.add(WorkAttributeConstant.SIMULATION_RESPONSE);
excludedAttributes.add(WorkAttributeConstant.DESCRIPTION);
excludedAttributes.add("BAM@START_MSGDEF");
excludedAttributes.add("BAM@FINISH_MSGDEF");
excludedAttributesForSpecificValues = new HashMap<>();
excludedAttributesForSpecificValues.put("DoNotNotifyCaller", "false");
excludedAttributesForSpecificValues.put("DO_LOGGING", "True");
tabularAttributes = new HashMap<>();
tabularAttributes.put("Notices",
Arrays.asList("Outcome", "Template", "Notifier Class(es)"));
tabularAttributes.put("Variables",
Arrays.asList("Variable", "ReferredAs", "Display", "Seq.", "Index"));
tabularAttributes.put("WAIT_EVENT_NAMES",
Arrays.asList("Event Name", "Completion Code", "Recurring"));
tabularAttributes.put("variables",
Arrays.asList("=", "SubProcess Variable", "Binding Expression"));
tabularAttributes.put("processmap",
Arrays.asList("=", "Logical Name", "Process Name", "Process Version"));
tabularAttributes.put("Bindings", Arrays.asList("=", "Variable", "LDAP Attribute"));
tabularAttributes.put("Parameters",
Arrays.asList("=", "Input Variable", "Binding Expression"));
textboxAttributes = new HashMap<>();
textboxAttributes.put("Rule", "Code");
textboxAttributes.put("Java", "Java");
textboxAttributes.put("PreScript", "Pre-Script");
textboxAttributes.put("PostScript", "Post-Script");
}
public String exportProcess(Process process, File outputDir) throws IOException {
new ActivityNodeSequencer(process).assignNodeSequenceIds();
StringBuilder sb = printPrologHtml("Process " + process.getName());
printProcessHtml(sb, 0, process, outputDir);
printEpilogHtml(sb);
return sb.toString();
}
private StringBuilder printPrologHtml(String title) {
StringBuilder sb = new StringBuilder();
sb.append("\n\n");
sb.append("").append(title).append(" \n");
sb.append("\n");
sb.append("\n\n");
return sb;
}
private void printProcessBodyHtml(StringBuilder sb, Process subproc) {
String tmp = null;
if (subproc.isEmbeddedProcess()) {
tmp = "Subprocess " + subproc.getAttribute(WorkAttributeConstant.LOGICAL_ID) + " - " + subproc.getName().replace('\n', ' ');
sb.append("").append(tmp).append("
\n");
}
String summary = subproc.getDescription();
if (summary != null && summary.length() > 0)
sb.append("").append(summary).append("");
String detail = subproc.getAttribute(WorkAttributeConstant.DOCUMENTATION);
if (detail != null && detail.length() > 0) {
printParagraphsHtml(sb, detail);
}
if (subproc.isEmbeddedProcess())
printAttributesHtml(sb, subproc.getAttributes());
sb.append(BR);
}
private void printParagraphsHtml(StringBuilder sb, String content) {
if (content == null || content.length() == 0)
return;
Parser parser = FlexmarkInstances.getParser(null);
HtmlRenderer renderer = FlexmarkInstances.getRenderer(null);
Node document = parser.parse(content);
sb.append(renderer.render(document));
}
private String escapeXml(String str) {
return str.replaceAll("&", "&").replaceAll("<", "<");
}
private void printAttributesHtml(StringBuilder sb, List attributes) {
if (attributes != null) {
List sortedAttrs = new ArrayList<>(attributes);
Collections.sort(sortedAttrs);
sb.append("Activity Attributes
\n");
sb.append("\n");
for (Attribute attr : sortedAttrs) {
String name = attr.getName();
String val = attr.getValue();
if (!excludeAttribute(name, val)) {
sb.append("- ");
if (tabularAttributes.containsKey(name)) {
sb.append(name + ":");
List
cols = new ArrayList<>(tabularAttributes.get(name));
char colDelim = ',';
if ("=".equals(cols.get(0))) {
colDelim = '=';
cols.remove(0);
}
String[] headers = cols.toArray(new String[0]);
List rows = Attribute.parseTable(escapeXml(val), colDelim, ';',
headers.length);
String[][] values = new String[headers.length][rows.size()];
for (int i = 0; i < headers.length; i++) {
for (int j = 0; j < rows.size(); j++) {
values[i][j] = rows.get(j)[i];
}
}
printTableHtml(sb, headers, values);
}
else if (textboxAttributes.containsKey(name)) {
sb.append(textboxAttributes.get(name) + ":");
printCodeBoxHtml(sb, escapeXml(val));
}
else {
sb.append(name + " = " + val);
}
sb.append(" ");
}
}
sb.append("
");
}
}
private boolean excludeAttribute(String name, String value) {
return (value == null || value.isEmpty() || excludedAttributes.contains(name)
|| value.equals(excludedAttributesForSpecificValues.get(name)));
}
private void printCodeBoxHtml(StringBuilder sb, String content) {
sb.append("").append(content)
.append("
");
}
private void printTableHtml(StringBuilder sb, String[] headers, String[][] values) {
String border = "border:1px solid black;";
String padding = "padding:3px;";
sb.append(
"\n");
sb.append("\n\n");
for (int i = 0; i < headers.length; i++) {
sb.append("").append(headers[i]).append(" \n");
}
sb.append(" \n");
sb.append("\n");
for (int i = 0; i < values[0].length; i++) {
sb.append("");
for (int j = 0; j < headers.length; j++) {
sb.append("").append(values[j][i])
.append(" ");
}
sb.append(" \n");
}
sb.append("\n");
sb.append("
\n");
}
private void printProcessHtml(StringBuilder sb, int chapter, Process process, File outputDir)
throws IOException {
sb.append("");
if (chapter > 0)
sb.append(Integer.toString(chapter)).append(". ");
sb.append("Workflow: \"").append(process.getName()).append("\"
\n");
// print image
printGraphHtml(sb, process, outputDir, chapter);
// print documentation text
sb.append(BR);
printProcessBodyHtml(sb, process);
for (Activity act : process.getActivitiesOrderBySeq()) {
printActivityHtml(sb, act);
}
for (Process subproc : process.getSubprocesses()) {
printProcessBodyHtml(sb, subproc);
for (Activity act : subproc.getActivitiesOrderBySeq()) {
printActivityHtml(sb, act);
}
}
List variables = process.getVariables();
if (variables != null && !variables.isEmpty()) {
sb.append("Process Variables
\n");
String[] headers = new String[] { "Name", "Type", "Mode" };
String[][] values = new String[3][variables.size()];
for (int i = 0; i < variables.size(); i++) {
Variable var = variables.get(i);
values[0][i] = var.getName();
values[1][i] = var.getType();
values[2][i] = var.getCategory();
}
printTableHtml(sb, headers, values);
}
}
private void printGraphHtml(StringBuilder sb, Process process, File outputDir,
int chapterNumber) throws IOException {
String imgfilename = process.getName() + "_" + process.getVersionString()
+ "_ch" + chapterNumber + ".jpg";
String imgfilepath = outputDir + "/" + imgfilename;
printImage(imgfilepath, process);
sb.append("
\n");
}
private void printActivityHtml(StringBuilder sb, Activity act) {
String tmp = ACTIVITY + act.getLogicalId() + ": \"" + act.getName().replace('\n', ' ') + "\"";
sb.append("").append(tmp).append("
\n");
String summary = act.getAttribute(WorkAttributeConstant.DESCRIPTION);
if (summary != null && summary.length() > 0)
sb.append("").append(summary).append("");
String detail = act.getAttribute(WorkAttributeConstant.DOCUMENTATION);
if (detail != null && detail.length() > 0) {
printParagraphsHtml(sb, detail);
}
printAttributesHtml(sb, act.getAttributes());
sb.append(BR);
}
public void printImage(String fileName, Process processVO) throws IOException {
ImageExportHelper imageHelper = new ImageExportHelper(project);
ImageIO.write(imageHelper.printImage(processVO), "jpeg", new File(fileName));
Runtime r = Runtime.getRuntime();
r.gc();
}
private void printEpilogHtml(StringBuilder sb) {
sb.append("\n");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy