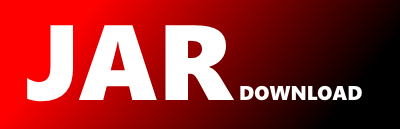
com.centurylink.mdw.services.user.ContextEmailRecipients Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mdw-services Show documentation
Show all versions of mdw-services Show documentation
MDW is a workflow framework specializing in microservice orchestration
/*
* Copyright (C) 2017 CenturyLink, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.centurylink.mdw.services.user;
import com.centurylink.mdw.dataaccess.DataAccessException;
import com.centurylink.mdw.model.user.Workgroup;
import com.centurylink.mdw.model.workflow.RuntimeContext;
import com.centurylink.mdw.service.data.user.UserGroupCache;
import com.centurylink.mdw.services.ServiceLocator;
import com.centurylink.mdw.util.ParseException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class ContextEmailRecipients {
private RuntimeContext context;
public ContextEmailRecipients(RuntimeContext context) {
this.context = context;
}
/**
* Default behavior returns the UNION of addresses specified via the expression attribute
* along with those specified by the designated workgroups attribute.
*/
public List getRecipients(String workgroupsAttr, String expressionAttr)
throws DataAccessException, ParseException {
List recipients = new ArrayList<>();
if (workgroupsAttr != null) {
List groupList = context.getAttributes().getList(workgroupsAttr);
for (String groupEmail : getGroupEmails(groupList)) {
if (!recipients.contains(groupEmail))
recipients.add(groupEmail);
}
}
if (expressionAttr != null) {
String expression = context.getAttribute(expressionAttr);
if (expression != null && !expression.isEmpty()) {
List expressionEmails;
if (expression.indexOf("${") >= 0)
expressionEmails = getRecipientsFromExpression(expression);
else
expressionEmails = Arrays.asList(expression.split(","));
for (String expressionEmail : expressionEmails) {
if (!recipients.contains(expressionEmail))
recipients.add(expressionEmail);
}
}
}
return recipients;
}
/**
* Must resolve to type of String or List.
* If a value corresponds to a group name, returns users in the group.
*/
public List getRecipientsFromExpression(String expression)
throws DataAccessException, ParseException {
Object recip = context.evaluate(expression);
if (recip == null) {
context.logWarn("Warning: Recipient expression '" + expression + "' resolves to null");
}
List recips = new ArrayList<>();
if (recip instanceof String) {
if (!((String)recip).isEmpty()) { // null list evaluates to empty string (why?)
Workgroup group = UserGroupCache.getWorkgroup((String)recip);
if (group != null)
recips.addAll(getGroupEmails(Arrays.asList(new String[]{group.getName()})));
else
recips.add((String)recip);
}
}
else if (recip instanceof List) {
for (Object email : (List)recip) {
Workgroup group = UserGroupCache.getWorkgroup(email.toString());
if (group != null) {
for (String groupEmail : getGroupEmails(Arrays.asList(new String[]{group.getName()}))) {
if (!recips.contains(groupEmail))
recips.add(groupEmail);
}
}
else {
if (!recips.contains(email))
recips.add(email.toString());
}
}
}
else {
throw new ParseException("Recipient expression resolved to unsupported type: " + expression + ": " + recip);
}
return recips;
}
public List getGroupEmails(List groups) throws DataAccessException {
return ServiceLocator.getUserServices().getWorkgroupEmails(groups);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy