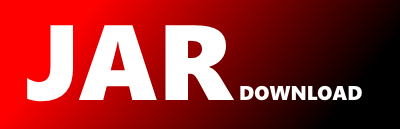
com.cerner.beadledom.jackson.JacksonModule Maven / Gradle / Ivy
package com.cerner.beadledom.jackson;
import com.fasterxml.jackson.databind.Module;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.google.inject.AbstractModule;
import com.google.inject.multibindings.Multibinder;
import com.google.inject.multibindings.MultibindingsScanner;
/**
* A Guice module that provides Jackson JSON serialization using {@link ObjectMapper}.
*
* With the module installed, you can add new {@link Module Jackson modules} to the
* {@link ObjectMapper} by adding bindings of {@link Module} using the Multibinder.
* Example:
*
* {@code
* class MyModule extends AbstractModule {
* {@literal @}Override
* protected void configure() {
* Multibinder<Module> jacksonModuleBinder = Multibinder
* .newSetBinder(binder(), Module.class);
* jacksonModuleBinder.addBinding().to(FoobarModule.class);
* }
* }}
*
*
* Provides:
*
* - {@link ObjectMapper}
*
*
* Installs:
*
* - {@link MultibindingsScanner}
*
*
* @author John Leacox
*/
public class JacksonModule extends AbstractModule {
@Override
protected void configure() {
// Empty multibindings for dependencies
Multibinder.newSetBinder(binder(), Module.class);
Multibinder.newSetBinder(binder(), SerializationFeatureFlag.class);
Multibinder.newSetBinder(binder(), DeserializationFeatureFlag.class);
Multibinder.newSetBinder(binder(), JsonGeneratorFeatureFlag.class);
Multibinder.newSetBinder(binder(), JsonParserFeatureFlag.class);
Multibinder.newSetBinder(binder(), MapperFeatureFlag.class);
/**
* MultibindingsScanner will scan all modules for methods with the annotations @ProvidesIntoMap,
* @ProvidesIntoSet, and @ProvidesIntoOptional.
*/
install(MultibindingsScanner.asModule());
bind(ObjectMapper.class).toProvider(ObjectMapperProvider.class).asEagerSingleton();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy