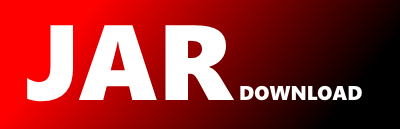
mesosphere.marathon.client.model.v2.Deployment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marathon-client Show documentation
Show all versions of marathon-client Show documentation
A Java API client for Mesosphere's Marathon.
package mesosphere.marathon.client.model.v2;
import java.util.Collection;
import java.util.List;
import mesosphere.client.common.ModelUtils;
public class Deployment {
private Collection affectedApps;
private String id;
private List steps;
private Collection currentActions;
private String version;
private Integer currentStep;
private Integer totalSteps;
public Collection getAffectedApps() {
return affectedApps;
}
public void setAffectedApps(Collection affectedApps) {
this.affectedApps = affectedApps;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public List getSteps() {
return steps;
}
public void setSteps(List steps) {
this.steps = steps;
}
public Collection getCurrentActions() {
return currentActions;
}
public void setCurrentActions(Collection currentActions) {
this.currentActions = currentActions;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public Integer getCurrentStep() {
return currentStep;
}
public void setCurrentStep(Integer currentStep) {
this.currentStep = currentStep;
}
public Integer getTotalSteps() {
return totalSteps;
}
public void setTotalSteps(Integer totalSteps) {
this.totalSteps = totalSteps;
}
public class Step {
private List actions;
public List getActions() {
return actions;
}
public void setActions(List actions) {
this.actions = actions;
}
@Override
public String toString() {
return ModelUtils.toString(this);
}
}
public class Action {
private String type;
private String app;
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getApp() {
return app;
}
public void setApp(String app) {
this.app = app;
}
@Override
public String toString() {
return ModelUtils.toString(this);
}
}
@Override
public String toString() {
return ModelUtils.toString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy