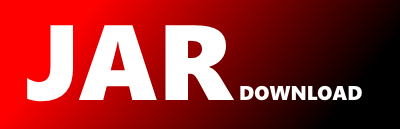
com.chain.sequence.api.Key Maven / Gradle / Ivy
The newest version!
package com.chain.sequence.api;
import com.chain.sequence.exception.*;
import com.chain.sequence.http.Client;
import java.net.MalformedURLException;
import java.util.*;
/**
* Keys are used to sign transactions.
*/
public class Key {
/**
* Unique identifier of the key, based on the public key material itself.
*/
public String id;
/**
* Unique, user-specified identifier of the key.
*/
public String alias;
/**
* Configuration object for creating keys.
*/
public static class Builder {
private String alias;
/**
* Sets a user-provided, unique identifier for the key.
* @param alias the new key's alias
* @return the updated builder
*/
public Builder setAlias(String alias) {
this.alias = alias;
return this;
}
/**
* Creates a key.
* @param client ledger API connection object
* @return a key object
* @throws ChainException
*/
public Key create(Client client) throws ChainException {
return client.request("create-key", this, Key.class);
}
}
public static class Page extends BasePage {}
public static class ItemIterable extends BaseItemIterable {
public ItemIterable(Client client, String path, Query nextQuery) {
super(client, path, nextQuery, Page.class);
}
}
public static class PageIterable extends BasePageIterable {
public PageIterable(Client client, String path, Query nextQuery) {
super(client, path, nextQuery, Page.class);
}
}
/**
* A builder class for querying keys in the ledger.
*/
public static class QueryBuilder extends BaseQueryBuilder {
/**
* Executes the query, returning a page of keys that match the query.
* @param client ledger API connection object
* @return a page of keys
* @throws ChainException
*/
public Page getPage(Client client) throws ChainException {
return client.request("list-keys", this.next, Page.class);
}
/**
* Executes the query, returning an iterable over keys that match the query.
* @param client ledger API connection object
* @return an iterable over keys
* @throws ChainException
*/
public ItemIterable getIterable(Client client) throws ChainException {
return new ItemIterable(client, "list-keys", this.next);
}
/**
* Executes the query, returning an iterable over pages of keys that match
* the query.
* @param client ledger API connection object
* @return an iterable over pages of keys
* @throws ChainException
*/
public PageIterable getPageIterable(Client client) throws ChainException {
return new PageIterable(client, "list-keys", this.next);
}
/**
* Specifies a list of aliases of keys to be queried.
* @param aliases a list of key aliases
* @return updated builder
*/
public QueryBuilder setAliases(List aliases) {
this.next.aliases = new ArrayList<>(aliases);
return this;
}
/**
* Adds an alias to the list of aliases of keys to be queried.
* @param alias a key alias
* @return updated builder
*/
public QueryBuilder addAlias(String alias) {
this.next.aliases.add(alias);
return this;
}
}
/**
* A composite key identifier, containing an alias and/or and ID.
*/
public static class Handle {
public String alias;
public String id;
/**
* Creates a new Handle from a Key.
* @param k a key
* @return a new handle based on the provided key
*/
public static Handle fromKey(Key k) {
Handle h = new Handle();
if (k.alias != null && !k.alias.equals("")) {
h.alias = k.alias;
}
if (k.id != null && !k.id.equals("")) {
h.id = k.id;
}
return h;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy