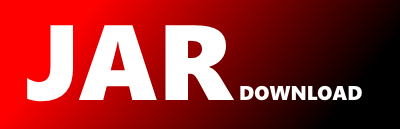
com.chain.sequence.api.UnspentOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sequence-sdk Show documentation
Show all versions of sequence-sdk Show documentation
The Official Java SDK for Chain Core Developer Edition
The newest version!
package com.chain.sequence.api;
import com.chain.sequence.exception.APIException;
import com.chain.sequence.exception.BadURLException;
import com.chain.sequence.exception.ChainException;
import com.chain.sequence.http.Client;
import com.chain.sequence.exception.ConnectivityException;
import com.chain.sequence.exception.JSONException;
import com.google.gson.annotations.SerializedName;
import java.util.Map;
public class UnspentOutput {
/**
* The ID of the output.
*/
@SerializedName("id")
public String id;
/**
* The type of action being taken on the output.
* Possible actions are "control_account", "control_program", and "retire".
*/
public String type;
/**
* The ID of the transaction in which the unspent output appears.
*/
@SerializedName("transaction_id")
public String transactionId;
/**
* The id of the asset being controlled.
*/
@SerializedName("asset_id")
public String assetId;
/**
* The alias of the asset being controlled.
*/
@SerializedName("asset_alias")
public String assetAlias;
/**
* The tags of the asset being controlled (possibly null).
*/
@SerializedName("asset_tags")
public Map assetTags;
/**
* The number of units of the asset being controlled.
*/
public long amount;
/**
* The id of the account controlling this output (possibly null if a control program is specified).
*/
@SerializedName("account_id")
public String accountId;
/**
* The alias of the account controlling this output (possibly null if a control program is specified).
*/
@SerializedName("account_alias")
public String accountAlias;
/**
* The tags associated with the account controlling this output (possibly null if a control program is specified).
*/
@SerializedName("account_tags")
public Map accountTags;
/**
* User specified, unstructured data embedded within an output (possibly null).
*/
@SerializedName("reference_data")
public Map referenceData;
public static class Page extends BasePage {}
public static class ItemIterable extends BaseItemIterable {
public ItemIterable(Client client, String path, Query nextQuery) {
super(client, path, nextQuery, Page.class);
}
}
public static class PageIterable extends BasePageIterable {
public PageIterable(Client client, String path, Query nextQuery) {
super(client, path, nextQuery, Page.class);
}
}
/**
* UnspentOutput.QueryBuilder utilizes the builder pattern to create {@link UnspentOutput} queries.
* The possible parameters for each query can be found on the {@link BaseQueryBuilder} class.
* All parameters are optional, and should be set to filter the results accordingly.
*/
public static class QueryBuilder extends BaseQueryBuilder {
/**
* Executes a query on the core's unspent outputs.
* @param client ledger API connection object
* @return a single page of unspent output objects
* @throws ChainException
*/
public Page getPage(Client client) throws ChainException {
return client.request("list-unspent-outputs", this.next, Page.class);
}
/**
* Executes a query on the core's unspent outputs.
* @param client ledger API connection object
* @return an iterable over unspent outputs matching the specified query
* @throws ChainException
*/
public ItemIterable getIterable(Client client) throws ChainException {
return new ItemIterable(client, "list-unspent-outputs", this.next);
}
/**
* Executes a query on the core's unspent outputs.
* @param client ledger API connection object
* @return an iterable over API pages matching the specified query
* @throws ChainException
*/
public PageIterable getPageIterable(Client client) throws ChainException {
return new PageIterable(client, "list-unspent-outputs", this.next);
}
/**
* Sets the latest timestamp for unspent outputs to be included in the results.
* @param timestampMS timestamp in milliseconds
* @return updated builder
*/
public QueryBuilder setTimestamp(long timestampMS) {
this.next.timestamp = timestampMS;
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy