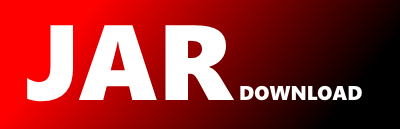
com.chargebee.internal.ResultBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chargebee-java Show documentation
Show all versions of chargebee-java Show documentation
Java client library for ChargeBee API
package com.chargebee.internal;
import com.chargebee.models.*;
import org.json.JSONException;
import org.json.JSONObject;
public class ResultBase {
private JSONObject jsonObj;
public ResultBase(JSONObject jsonObj) {
this.jsonObj = jsonObj;
}
public Subscription subscription() {
return (Subscription)get("subscription");
}
public Customer customer() {
return (Customer)get("customer");
}
public Card card() {
return (Card)get("card");
}
public Invoice invoice() {
return (Invoice)get("invoice");
}
public Transaction transaction() {
return (Transaction)get("transaction");
}
public HostedPage hostedPage() {
return (HostedPage)get("hosted_page");
}
public Estimate estimate() {
return (Estimate)get("estimate");
}
public Plan plan() {
return (Plan)get("plan");
}
public Addon addon() {
return (Addon)get("addon");
}
public Coupon coupon() {
return (Coupon)get("coupon");
}
public CouponCode couponCode() {
return (CouponCode)get("coupon_code");
}
public Address address() {
return (Address)get("address");
}
public Event event() {
return (Event)get("event");
}
public Comment comment() {
return (Comment)get("comment");
}
public Download download() {
return (Download)get("download");
}
private Resource get(String key) {
JSONObject modelJson = jsonObj.optJSONObject(key);
if(modelJson == null) {
return null;
}
Class modelClaz = ClazzUtil.getClaz(key);
return ClazzUtil.createInstance(modelClaz, modelJson);
}
@Override
public String toString() {
try {
return jsonObj.toString(2);
} catch (JSONException ex) {
throw new RuntimeException(ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy