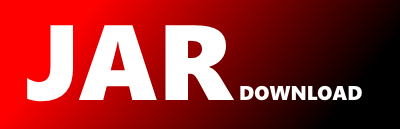
chaschev.lang.Lists2 Maven / Gradle / Ivy
package chaschev.lang;
import com.google.common.base.Function;
import com.google.common.base.Predicates;
import com.google.common.base.Supplier;
import com.google.common.collect.Iterables;
import com.google.common.collect.Lists;
import java.util.AbstractList;
import java.util.Collections;
import java.util.List;
/**
* @author Andrey Chaschev [email protected]
*/
public class Lists2 {
public static List projectField(List fromList, Class elClass, Class fieldClass, String name) {
Function field = (Function) Functions2.field(name);
return Lists.transform(fromList, field);
}
public static List projectMethod(List fromList, Class elClass, Class methodClass, String name) {
Function method = (Function) Functions2.method(name);
return Lists.transform(fromList, method);
}
public static List projectField(List fromList, String name) {
F nonNull = Iterables.find(fromList, Predicates.notNull(), null);
if(nonNull == null){
if(fromList.isEmpty()){
return Collections.emptyList();
}else{
return Collections.nCopies(fromList.size(), null);
}
}
Class aClass = nonNull.getClass();
return projectField(fromList, aClass, null, name);
}
public static List projectMethod(List fromList, String name) {
F nonNull = Iterables.find(fromList, Predicates.notNull(), null);
if(nonNull == null){
if(fromList.isEmpty()){
return Collections.emptyList();
}else{
return Collections.nCopies(fromList.size(), null);
}
}
Class aClass = nonNull.getClass();
return projectMethod(fromList, aClass, null, name);
}
public static List removeDupsInSortedList(List list) {
int w = 0;
for (int r = 0, listSize = list.size(); r < listSize; r++) {
if (r == 0) {
w++;
continue;
}
if (!list.get(r).equals(list.get(r - 1))) {
list.set(w, list.get(r));
w++;
}
}
for (int last = list.size() - 1; last >= w; last--) {
list.remove(last);
}
return list;
}
public static List newFilledArrayList(int size, T value) {
List list = Lists.newArrayListWithExpectedSize(size);
for (int i = 0; i < size; i++) {
list.add(value);
}
return list;
}
public static List nInstances(int n, Supplier supplier){
List list = Lists.newArrayListWithCapacity(n);
for(int i = 0; i < n; i++){
list.add(supplier.get());
}
return list;
}
public static List computingList(final int n, final Function function){
return new AbstractList() {
@Override
public T get(int index) {
return function.apply(index);
}
@Override
public int size() {
return n;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy