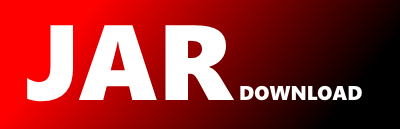
com.bethecoder.table.impl.CollectionASCIITableAware Maven / Gradle / Ivy
/**
* Copyright (C) 2011 K Venkata Sudhakar
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.bethecoder.table.impl;
import com.bethecoder.table.ASCIITableHeader;
import com.bethecoder.table.spec.IASCIITableAware;
import java.lang.reflect.Method;
import java.math.BigDecimal;
import java.text.DecimalFormat;
import java.util.*;
/**
* This class is useful to extract the header and row data from
* a list of java beans.
*
* @author K Venkata Sudhakar ([email protected])
* @version 1.0
*
*/
public class CollectionASCIITableAware implements IASCIITableAware {
private List headers = null;
private List> data = null;
public CollectionASCIITableAware(List objList, String ... properties) {
this(objList, Arrays.asList(properties), Arrays.asList(properties));
}
public CollectionASCIITableAware(List objList, List properties, List title) {
if (objList != null && !objList.isEmpty() && properties != null && !properties.isEmpty()) {
//Populate header
String header = null;
headers = new ArrayList(properties.size());
for (int i = 0 ; i < properties.size() ; i ++) {
header = (i < title.size()) ? title.get(i) : properties.get(i);
headers.add(new ASCIITableHeader(String.valueOf(header).toUpperCase()));
}
//Populate data
data = new ArrayList>();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy