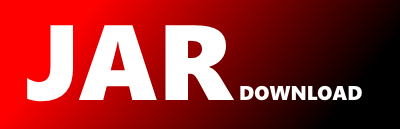
com.checkout.ApiClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of checkout-sdk-java Show documentation
Show all versions of checkout-sdk-java Show documentation
Checkout SDK for Java https://checkout.com
package com.checkout;
import com.checkout.common.AbstractFileRequest;
import com.checkout.common.CheckoutUtils;
import com.google.gson.reflect.TypeToken;
import org.apache.commons.lang3.StringUtils;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.IOException;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.nio.file.Files;
import java.nio.file.StandardCopyOption;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import static com.checkout.ClientOperation.DELETE;
import static com.checkout.ClientOperation.GET;
import static com.checkout.ClientOperation.PATCH;
import static com.checkout.ClientOperation.POST;
import static com.checkout.ClientOperation.PUT;
import static com.checkout.ClientOperation.QUERY;
import static com.checkout.common.CheckoutUtils.validateParams;
public class ApiClientImpl implements ApiClient {
private static final String AUTHORIZATION = "authorization";
private static final String PATH = "path";
private final Serializer serializer;
private final Transport transport;
public ApiClientImpl(final CheckoutConfiguration configuration, final UriStrategy uriStrategy) {
this.serializer = new GsonSerializer();
this.transport = new ApacheHttpClientTransport(uriStrategy.getUri(), configuration.getHttpClientBuilder(), configuration.getExecutor());
}
@Override
public CompletableFuture getAsync(final String path, final SdkAuthorization authorization, final Class responseType) {
validateParams(PATH, path, AUTHORIZATION, authorization);
return sendRequestAsync(GET, path, authorization, null, null, responseType);
}
@Override
public CompletableFuture getAsync(final String path, final SdkAuthorization authorization, final Type responseType) {
validateParams(PATH, path, AUTHORIZATION, authorization);
return sendRequestAsync(GET, path, authorization, null, null, responseType);
}
@Override
public CompletableFuture putAsync(final String path, final SdkAuthorization authorization, final Class responseType, final Object request) {
validateParams(PATH, path, AUTHORIZATION, authorization);
return sendRequestAsync(PUT, path, authorization, request, null, responseType);
}
@Override
public CompletableFuture patchAsync(final String path, final SdkAuthorization authorization, final Class responseType, final Object request, final String idempotencyKey) {
validateParams(PATH, path, AUTHORIZATION, authorization);
return sendRequestAsync(PATCH, path, authorization, request, idempotencyKey, responseType);
}
@Override
public CompletableFuture patchAsync(final String path, final SdkAuthorization authorization, final Type type, final Object request, final String idempotencyKey) {
validateParams(PATH, path, AUTHORIZATION, authorization, "type", type, "request", request);
return sendRequestAsync(PATCH, path, authorization, request, idempotencyKey, type);
}
@Override
public CompletableFuture postAsync(final String path, final SdkAuthorization authorization, final Class responseType, final Object request, final String idempotencyKey) {
validateParams(PATH, path, AUTHORIZATION, authorization);
return sendRequestAsync(POST, path, authorization, request, idempotencyKey, responseType);
}
@Override
public CompletableFuture postAsync(final String path, final SdkAuthorization authorization, final Type responseType, final Object request, final String idempotencyKey) {
validateParams(PATH, path, AUTHORIZATION, authorization);
return sendRequestAsync(POST, path, authorization, request, idempotencyKey, responseType);
}
@Override
public CompletableFuture deleteAsync(final String path, final SdkAuthorization authorization) {
validateParams(PATH, path, AUTHORIZATION, authorization);
return sendRequestAsync(DELETE, path, authorization, null, null, EmptyResponse.class);
}
@Override
public CompletableFuture deleteAsync(String path, SdkAuthorization authorization, Class responseType) {
validateParams(PATH, path, AUTHORIZATION, authorization);
return sendRequestAsync(DELETE, path, authorization, null, null, responseType);
}
@Override
public CompletableFuture extends HttpMetadata> postAsync(final String path, final SdkAuthorization authorization, final Map> resultTypeMappings, final Object request, final String idempotencyKey) {
validateParams(PATH, path, AUTHORIZATION, authorization, "resultTypeMappings", resultTypeMappings);
return transport.invoke(POST, path, authorization, serializer.toJson(request), idempotencyKey, null)
.thenApply(this::errorCheck)
.thenApply(response -> {
final Class extends HttpMetadata> responseType = resultTypeMappings.get(response.getStatusCode());
if (responseType == null) {
throw new IllegalStateException("The status code " + response.getStatusCode() + " is not mapped to a result type");
}
return deserialize(response, responseType);
});
}
@Override
public CompletableFuture queryAsync(final String path,
final SdkAuthorization authorization,
final Object filter,
final Class responseType) {
validateParams(PATH, path, AUTHORIZATION, authorization, "filter", filter);
final Map params = serializer.fromJson(serializer.toJson(filter),
new TypeToken
© 2015 - 2024 Weber Informatics LLC | Privacy Policy