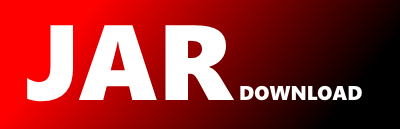
com.checkout.accounts.AccountsClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of checkout-sdk-java Show documentation
Show all versions of checkout-sdk-java Show documentation
Checkout SDK for Java https://checkout.com
package com.checkout.accounts;
import com.checkout.AbstractClient;
import com.checkout.ApiClient;
import com.checkout.CheckoutConfiguration;
import com.checkout.EmptyResponse;
import com.checkout.SdkAuthorizationType;
import com.checkout.accounts.payout.schedule.request.UpdateScheduleRequest;
import com.checkout.accounts.payout.schedule.response.GetScheduleResponse;
import com.checkout.accounts.payout.schedule.response.VoidResponse;
import com.checkout.common.Currency;
import com.checkout.common.IdResponse;
import java.util.EnumMap;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import static com.checkout.common.CheckoutUtils.validateParams;
public class AccountsClientImpl extends AbstractClient implements AccountsClient {
private static final String ACCOUNTS_PATH = "accounts";
private static final String ENTITIES_PATH = "entities";
private static final String INSTRUMENTS_PATH = "instruments";
private static final String FILES_PATH = "files";
private static final String PAYOUT_SCHEDULES_PATH = "payout-schedules";
private static final String PAYMENT_INSTRUMENTS_PATH = "payment-instruments";
private final ApiClient filesClient;
public AccountsClientImpl(final ApiClient apiClient,
final ApiClient filesClient,
final CheckoutConfiguration configuration) {
super(apiClient, configuration, SdkAuthorizationType.SECRET_KEY_OR_OAUTH);
this.filesClient = filesClient;
}
@Override
public CompletableFuture submitFile(final AccountsFileRequest accountsFileRequest) {
validateParams("accountsFileRequest", accountsFileRequest);
return filesClient.submitFileAsync(
FILES_PATH,
sdkAuthorization(),
accountsFileRequest,
IdResponse.class);
}
@Override
public CompletableFuture createEntity(final OnboardEntityRequest entityRequest) {
validateParams("entityRequest", entityRequest);
return apiClient.postAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH),
sdkAuthorization(),
OnboardEntityResponse.class,
entityRequest,
null);
}
@Override
public CompletableFuture retrievePaymentInstrumentDetails(final String entityId, final String paymentInstrumentId) {
validateParams("entityId", entityId, "paymentInstrumentId", paymentInstrumentId);
return apiClient.getAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH, entityId, PAYMENT_INSTRUMENTS_PATH, paymentInstrumentId),
sdkAuthorization(),
PaymentInstrumentDetailsResponse.class);
}
@Override
public CompletableFuture getEntity(final String entityId) {
validateParams("entityId", entityId);
return apiClient.getAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH, entityId),
sdkAuthorization(),
OnboardEntityDetailsResponse.class);
}
@Override
public CompletableFuture updateEntity(final OnboardEntityRequest entityRequest, final String entityId) {
validateParams("entityRequest", entityRequest, "entityId", entityId);
return apiClient.putAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH, entityId),
sdkAuthorization(),
OnboardEntityResponse.class,
entityRequest);
}
@Override
public CompletableFuture createPaymentInstrument(final AccountsPaymentInstrument accountsPaymentInstrument, final String entityId) {
validateParams("accountsPaymentInstrument", accountsPaymentInstrument, "entityId", entityId);
return apiClient.postAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH, entityId, INSTRUMENTS_PATH),
sdkAuthorization(),
EmptyResponse.class,
accountsPaymentInstrument,
null);
}
@Override
public CompletableFuture createPaymentInstrument(final String entityId, final PaymentInstrumentRequest paymentInstrumentRequest) {
validateParams("entityId", entityId, "paymentInstrumentRequest", paymentInstrumentRequest);
return apiClient.postAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH, entityId, PAYMENT_INSTRUMENTS_PATH),
sdkAuthorization(),
IdResponse.class,
paymentInstrumentRequest,
null
);
}
@Override
public CompletableFuture updatePaymentInstrumentDetails(final String entityId,
final String instrumentId,
final UpdatePaymentInstrumentRequest updatePaymentInstrumentRequest) {
validateParams("entityId", entityId, "instrumentId", instrumentId, "updatePaymentInstrumentRequest", updatePaymentInstrumentRequest);
return apiClient.patchAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH, entityId, PAYMENT_INSTRUMENTS_PATH, instrumentId),
sdkAuthorization(),
IdResponse.class,
updatePaymentInstrumentRequest,
null
);
}
@Override
public CompletableFuture queryPaymentInstruments(final String entityId, final PaymentInstrumentsQuery query) {
validateParams("entityId", entityId, "query", query);
return apiClient.queryAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH, entityId, PAYMENT_INSTRUMENTS_PATH),
sdkAuthorization(),
query,
PaymentInstrumentQueryResponse.class
);
}
@Override
public CompletableFuture retrievePayoutSchedule(final String entityId) {
validateParams("entityId", entityId);
return apiClient.getAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH, entityId, PAYOUT_SCHEDULES_PATH),
sdkAuthorization(),
GetScheduleResponse.class);
}
@Override
public CompletableFuture updatePayoutSchedule(final String entityId, final Currency currency, final UpdateScheduleRequest updateScheduleRequest) {
validateParams("entityId", entityId, "currency", currency, "updateScheduleRequest", updateScheduleRequest);
final Map request = new EnumMap<>(Currency.class);
request.put(currency, updateScheduleRequest);
return apiClient.putAsync(
buildPath(ACCOUNTS_PATH, ENTITIES_PATH, entityId, PAYOUT_SCHEDULES_PATH),
sdkAuthorization(),
VoidResponse.class,
request);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy