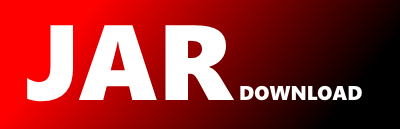
com.chimpcentral.archive.Compiler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of logger Show documentation
Show all versions of logger Show documentation
Logger to create HTML and TEXT logs
package com.chimpcentral.archive;
import java.io.IOException;
import java.util.List;
import com.chimpcentral.io.FileStatus;
import com.chimpcentral.io.FlatFile;
public class Compiler {
private Logger logger = null;
private LoggerInfo loggerInfo = null;
private ContentHelper fileHelper = null;
private FlatFile logFile = null;
public Compiler(Logger logger) {
this.logger = logger;
this.loggerInfo = this.logger.getLoggerInfo();
this.fileHelper = new ContentHelper(this.loggerInfo.getResourcesDir());
}
private void createLogFile(String filepath) {
try {
this.logFile = new FlatFile(filepath, FileStatus.NEW);
} catch (IOException e) {
e.printStackTrace();
}
}
private void appendContentToLogFile(String content) {
try {
this.logFile.appendContent(content);
} catch (IOException e) {
e.printStackTrace();
}
}
private StringBuilder getTableHeader() {
return new StringBuilder()
.append("")
.append("")
.append("")
.append("log info ")
.append("time ")
.append("message ")
.append(" ")
.append("");
}
private StringBuilder getTableBodyStart() {
return new StringBuilder().append("");
}
private StringBuilder getTableBodyEnd() {
return new StringBuilder().append("
");
}
private StringBuilder getLogContainer() {
return new StringBuilder().append("");
}
private void processMainLog(Log log) {
String content = log.getContent().toString();
if (content != null && !content.equals("")) {
appendContentToLogFile(getTableHeader().toString());
appendContentToLogFile(getTableBodyStart().toString());
appendContentToLogFile(content);
appendContentToLogFile(getTableBodyEnd().toString());
appendContentToLogFile("");//this closes the main text
}
if (log.hasNodes()) processLogs(log.getNodes());
}
private void processLog(Log log) {
String content = log.getContent().toString();
appendContentToLogFile(getLogContainer().toString());
String logStartContent = this.fileHelper.getNodeLogStartFileContent().toString().replaceAll("", log.getName().replaceAll(" ", "_"));
appendContentToLogFile(logStartContent);
appendContentToLogFile(getTableHeader().toString());
appendContentToLogFile(getTableBodyStart().toString());
appendContentToLogFile(content);
appendContentToLogFile(getTableBodyEnd().toString());
appendContentToLogFile(this.fileHelper.getNodeLogEndFileContent().toString());
if (log.hasNodes()) processLogs(log.getNodes());
else appendContentToLogFile("