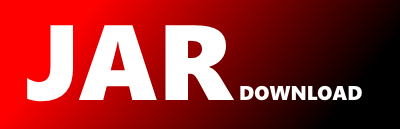
com.zx.sms.codec.smpp.SMPPMessageCodec Maven / Gradle / Ivy
package com.zx.sms.codec.smpp;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.handler.codec.MessageToMessageCodec;
import java.util.List;
import com.zx.sms.codec.smpp.msg.Pdu;
public class SMPPMessageCodec extends MessageToMessageCodec {
private static final PduTranscoder transcoder = new DefaultPduTranscoder(new DefaultPduTranscoderContext());
@Override
protected void encode(ChannelHandlerContext ctx, Pdu msg, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy