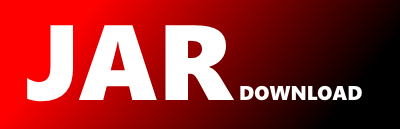
org.adoptopenjdk.jitwatch.ui.optimizedvcall.VCallTableBuilder Maven / Gradle / Ivy
/*
* Copyright (c) 2013-2016 Chris Newland.
* Licensed under https://github.com/AdoptOpenJDK/jitwatch/blob/master/LICENSE-BSD
* Instructions: https://github.com/AdoptOpenJDK/jitwatch/wiki
*/
package org.adoptopenjdk.jitwatch.ui.optimizedvcall;
import java.util.ArrayList;
import java.util.List;
import javafx.collections.FXCollections;
import javafx.event.EventHandler;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.input.MouseEvent;
import org.adoptopenjdk.jitwatch.model.IMetaMember;
import org.adoptopenjdk.jitwatch.optimizedvcall.OptimizedVirtualCall;
import org.adoptopenjdk.jitwatch.ui.main.IStageAccessProxy;
public class VCallTableBuilder
{
public static TableView buildTable(final IStageAccessProxy proxy, List vCalls)
{
final TableView tableView = new TableView<>();
TableColumn colCallerClass = new TableColumn("Caller Class");
colCallerClass.setCellValueFactory(new PropertyValueFactory("callerClass"));
TableColumn colCallerMember = new TableColumn("Method");
colCallerMember.setCellValueFactory(new PropertyValueFactory("callerMember"));
TableColumn colCallerLine = new TableColumn("Source Line");
colCallerLine.setCellValueFactory(new PropertyValueFactory("callerSourceLine"));
TableColumn colCallerBytecode = new TableColumn("BCI");
colCallerBytecode.setCellValueFactory(new PropertyValueFactory("callerBCI"));
TableColumn colInvokeType = new TableColumn("Invoke");
colInvokeType.setCellValueFactory(new PropertyValueFactory("invokeType"));
tableView.getColumns().add(colCallerClass);
tableView.getColumns().add(colCallerMember);
tableView.getColumns().add(colCallerLine);
tableView.getColumns().add(colCallerBytecode);
tableView.getColumns().add(colInvokeType);
TableColumn colCalleeClass = new TableColumn("Callee Class");
colCalleeClass.setCellValueFactory(new PropertyValueFactory("calleeClass"));
TableColumn colCalleeMember = new TableColumn("Method");
colCalleeMember.setCellValueFactory(new PropertyValueFactory("calleeMember"));
tableView.getColumns().add(colCalleeClass);
tableView.getColumns().add(colCalleeMember);
tableView.setOnMouseClicked(new EventHandler()
{
@Override
public void handle(MouseEvent event)
{
VCallRow selected = tableView.getSelectionModel().getSelectedItem();
if (selected != null)
{
IMetaMember callingMember = selected.getCaller();
proxy.openTriView(callingMember, true, selected.getCallerBCI());
}
}
});
List rowList = new ArrayList<>();
if (vCalls != null)
{
for (OptimizedVirtualCall vCall : vCalls)
{
VCallRow row = new VCallRow(vCall);
rowList.add(row);
}
}
tableView.setItems(FXCollections.observableArrayList(rowList));
return tableView;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy