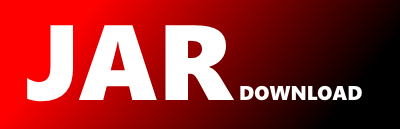
org.adoptopenjdk.jitwatch.ui.report.elidedlock.ElidedLockRowBuilder Maven / Gradle / Ivy
/*
* Copyright (c) 2017 Chris Newland.
* Licensed under https://github.com/AdoptOpenJDK/jitwatch/blob/master/LICENSE-BSD
* Instructions: https://github.com/AdoptOpenJDK/jitwatch/wiki
*/
package org.adoptopenjdk.jitwatch.ui.report.elidedlock;
import org.adoptopenjdk.jitwatch.report.Report;
import org.adoptopenjdk.jitwatch.ui.report.IReportRowBean;
import org.adoptopenjdk.jitwatch.ui.report.cell.LinkedBCICell;
import org.adoptopenjdk.jitwatch.ui.report.cell.TextTableCell;
import javafx.collections.ObservableList;
import javafx.scene.control.TableCell;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.util.Callback;
public final class ElidedLockRowBuilder
{
private ElidedLockRowBuilder()
{
}
public static TableView buildTableSuggestion(ObservableList rows)
{
TableView tv = new TableView<>();
TableColumn metaClass = new TableColumn("Class");
metaClass.setCellValueFactory(new PropertyValueFactory("metaClass"));
metaClass.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new TextTableCell();
}
});
TableColumn member = new TableColumn("Member");
member.setCellValueFactory(new PropertyValueFactory("member"));
member.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new TextTableCell();
}
});
TableColumn compilation = new TableColumn("Compilation");
compilation.setCellValueFactory(new PropertyValueFactory("compilation"));
compilation.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new TextTableCell();
}
});
TableColumn viewBCI = new TableColumn("BCI");
viewBCI.setCellValueFactory(new PropertyValueFactory("report"));
viewBCI.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new LinkedBCICell();
}
});
TableColumn directOrInline = new TableColumn("How");
directOrInline.setCellValueFactory(new PropertyValueFactory("kind"));
directOrInline.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new TextTableCell();
}
});
TableColumn elisionKind = new TableColumn("Elision Kind");
elisionKind.setCellValueFactory(new PropertyValueFactory("elisionKind"));
elisionKind.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new TextTableCell();
}
});
metaClass.prefWidthProperty().bind(tv.widthProperty().multiply(0.2));
member.prefWidthProperty().bind(tv.widthProperty().multiply(0.2));
compilation.prefWidthProperty().bind(tv.widthProperty().multiply(0.2));
viewBCI.prefWidthProperty().bind(tv.widthProperty().multiply(0.12));
directOrInline.prefWidthProperty().bind(tv.widthProperty().multiply(0.1));
elisionKind.prefWidthProperty().bind(tv.widthProperty().multiply(0.18));
tv.getColumns().add(metaClass);
tv.getColumns().add(member);
tv.getColumns().add(compilation);
tv.getColumns().add(viewBCI);
tv.getColumns().add(directOrInline);
tv.getColumns().add(elisionKind);
tv.setItems(rows);
return tv;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy