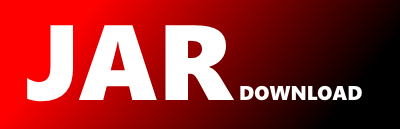
org.adoptopenjdk.jitwatch.ui.report.suggestion.SuggestionRowBuilder Maven / Gradle / Ivy
/*
* Copyright (c) 2013-2016 Chris Newland.
* Licensed under https://github.com/AdoptOpenJDK/jitwatch/blob/master/LICENSE-BSD
* Instructions: https://github.com/AdoptOpenJDK/jitwatch/wiki
*/
package org.adoptopenjdk.jitwatch.ui.report.suggestion;
import org.adoptopenjdk.jitwatch.report.Report;
import org.adoptopenjdk.jitwatch.ui.report.IReportRowBean;
import org.adoptopenjdk.jitwatch.ui.report.cell.IntegerTableCell;
import org.adoptopenjdk.jitwatch.ui.report.cell.MemberTableCell;
import org.adoptopenjdk.jitwatch.ui.report.cell.TextWrapTableCell;
import org.adoptopenjdk.jitwatch.ui.report.cell.TextTableCell;
import javafx.collections.ObservableList;
import javafx.scene.control.TableCell;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TableView;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.util.Callback;
public final class SuggestionRowBuilder
{
private SuggestionRowBuilder()
{
}
public static TableView buildTableSuggestion(ObservableList rows)
{
TableView tv = new TableView<>();
TableColumn score = new TableColumn("Score");
score.setCellValueFactory(new PropertyValueFactory("score"));
score.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new IntegerTableCell();
}
});
TableColumn type = new TableColumn("Type");
type.setCellValueFactory(new PropertyValueFactory("type"));
type.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new TextTableCell();
}
});
TableColumn caller = new TableColumn("Caller");
caller.setCellValueFactory(new PropertyValueFactory("report"));
caller.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new MemberTableCell();
}
});
TableColumn suggestion = new TableColumn("Suggestion");
suggestion.setCellValueFactory(new PropertyValueFactory("text"));
suggestion.setCellFactory(new Callback, TableCell>()
{
@Override
public TableCell call(TableColumn col)
{
return new TextWrapTableCell();
}
});
score.prefWidthProperty().bind(tv.widthProperty().multiply(0.05));
type.prefWidthProperty().bind(tv.widthProperty().multiply(0.1));
caller.prefWidthProperty().bind(tv.widthProperty().multiply(0.35));
suggestion.prefWidthProperty().bind(tv.widthProperty().multiply(0.50));
tv.getColumns().add(score);
tv.getColumns().add(type);
tv.getColumns().add(caller);
tv.getColumns().add(suggestion);
tv.setItems(rows);
return tv;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy