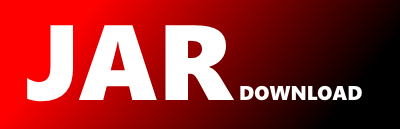
com.ch.email.service.SMTPEmailService Maven / Gradle / Ivy
The newest version!
package com.ch.email.service;
import java.io.File;
import java.util.Properties;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.activation.DataHandler;
import javax.activation.DataSource;
import javax.activation.FileDataSource;
import javax.mail.BodyPart;
import javax.mail.Message;
import javax.mail.Multipart;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import org.apache.commons.lang3.StringUtils;
import com.ch.config.ConfigProperties;
import com.ch.email.config.EmailConfiguration;
import com.ch.email.exception.EmailServiceException;
import com.ch.exception.PropertyNotFoundException;
public class SMTPEmailService implements EmailService {
private static final Logger LOGGER = Logger.getLogger(SMTPEmailService.class.getName());
// Fields for email content and properties
private String emailBody;
private String emailSubject;
private File emailAttachment;
private String emailDownloadableLink;
private EmailConfiguration config;
public SMTPEmailService(ConfigProperties configProperties) {
try {
config = convert(configProperties);
LOGGER.info("Initializing EmailConfiguration");
} catch (Exception e) {
LOGGER.log(Level.SEVERE, "Unable to EmailConfiguration ", e);
}
}
public EmailConfiguration getConfig() {
return config;
}
public void setConfig(EmailConfiguration config) {
this.config = config;
}
private static EmailConfiguration convert(ConfigProperties config) throws EmailServiceException {
EmailConfiguration configuration = new EmailConfiguration();
String isSmtpAuthEnabled = null;
try {
isSmtpAuthEnabled = config.getProperty(com.ch.email.constants.EmailConstants.MAIL_SMTP_AUTH_ENABLE);
} catch (PropertyNotFoundException e) {
e.printStackTrace();
isSmtpAuthEnabled = "false";
}
configuration.setSmtpAuthEnable(Boolean.parseBoolean(isSmtpAuthEnabled));
String isSmtpSSLEnable = null;
try {
isSmtpSSLEnable = config.getProperty(com.ch.email.constants.EmailConstants.MAIL_SMTP_SSL_ENABLE);
} catch (PropertyNotFoundException e) {
isSmtpSSLEnable = "false";
}
configuration.setSmtpSSLEnable(Boolean.parseBoolean(isSmtpSSLEnable));
String isSmtpStartTTL = null;
try {
isSmtpStartTTL = config.getProperty(com.ch.email.constants.EmailConstants.MAIL_SMTP_STARTTLS_ENABLE);
} catch (PropertyNotFoundException e) {
isSmtpStartTTL = "false";
}
configuration.setSmtpStartTLSEnable(Boolean.parseBoolean(isSmtpStartTTL));
String sessionDebug = null;
try {
isSmtpStartTTL = config.getProperty(com.ch.email.constants.EmailConstants.SESSION_DEBUG_ENABLE);
} catch (PropertyNotFoundException e) {
sessionDebug = "false";
}
configuration.setSessionDebug(Boolean.parseBoolean(sessionDebug));
try {
configuration.setSmtpHost(config.getProperty(com.ch.email.constants.EmailConstants.MAIL_SMTP_HOST));
configuration.setSmtpPort(config.getProperty(com.ch.email.constants.EmailConstants.MAIL_SMTP_PORT));
configuration.setFromAddress(config.getProperty(com.ch.email.constants.EmailConstants.FROM_ADDRESS));
configuration.setToAddresses(config.getProperty(com.ch.email.constants.EmailConstants.TO_RECIPIENTS));
if (configuration.isSmtpAuthEnable()) {
configuration.setUser(config.getProperty(com.ch.email.constants.EmailConstants.USER));
configuration.setPassword(config.getProperty(com.ch.email.constants.EmailConstants.PASSWORD));
}
} catch (PropertyNotFoundException e) {
throw new EmailServiceException("email configuration not available ", e);
}
return configuration;
}
private static Properties getProperties(EmailConfiguration config) throws EmailServiceException {
Properties props = new Properties();
props.setProperty("mail.smtp.host", config.getSmtpHost());
props.setProperty("mail.smtp.port", config.getSmtpPort());
if (config.isSmtpAuthEnable()) {
props.setProperty("mail.smtp.auth", "true");
}
if (config.isSmtpSSLEnable()) {
props.setProperty("mail.smtp.ssl.enable", "true");
}
if (config.isSmtpStartTLSEnable()) {
props.setProperty("mail.smtp.starttls.enable", "true");
}
return props;
}
private static Session getSession(EmailConfiguration config) throws EmailServiceException {
Session session = null;
Properties props = getProperties(config);
if (config.isSmtpAuthEnable()) {
session = Session.getInstance(props, new javax.mail.Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(config.getUser(), config.getPassword());
}
});
} else {
session = Session.getInstance(props, null);
}
return session;
}
@Override
public void sendEmail() throws EmailServiceException {
if (emailAttachment != null && emailAttachment.exists()) {
long fileByteSize = emailAttachment.length();
long oneMBBytes = 1000000;
long thresholdBytes = oneMBBytes * 10;
if (fileByteSize > thresholdBytes) {
LOGGER.warning("Email Attachment is too big to send as attachment ...");
LOGGER.info("Emailing TestSummary without attachment");
emailNoAttachment();
} else {
LOGGER.info("Emailing TestSummary with attachment");
sendEmailWithAttachment();
}
} else {
LOGGER.info("Attachment not available");
LOGGER.info("Emailing TestSummary without attachment");
emailNoAttachment();
}
}
public void emailNoAttachment() throws EmailServiceException {
if (StringUtils.isNotBlank(emailSubject) && StringUtils.isNotBlank(emailBody)) {
try {
Session session = getSession(config);
if (config.isSessionDebug()) {
session.setDebug(true);
}
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(config.getFromAddress()));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(config.getToAddresses()));
if (StringUtils.isNotBlank(config.getCcAddresses())) {
message.setRecipients(Message.RecipientType.CC, InternetAddress.parse(config.getCcAddresses()));
}
if (StringUtils.isNotBlank(config.getBccAddresses())) {
message.setRecipients(Message.RecipientType.BCC, InternetAddress.parse(config.getBccAddresses()));
}
message.setSubject(emailSubject);
BodyPart messageBodyPart = new MimeBodyPart();
messageBodyPart.setContent(emailBody, "text/html;charset=UTF-8");
Multipart multipart = new MimeMultipart();
multipart.addBodyPart(messageBodyPart);
message.setContent(multipart);
Transport.send(message);
LOGGER.info("Sent message successfully....");
} catch (Exception e) {
LOGGER.log(Level.SEVERE, "Exception EmailService emailNoAttachment()", e);
throw new EmailServiceException("Error sending email", e);
}
} else {
LOGGER.warning("Email subject or body is empty. Email not sent.");
}
}
public void sendEmailWithAttachment() throws EmailServiceException {
if (StringUtils.isNotBlank(emailSubject) && StringUtils.isNotBlank(emailBody)) {
try {
Session session = getSession(config);
if (config.isSessionDebug()) {
session.setDebug(true);
}
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(config.getFromAddress()));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(config.getToAddresses()));
if (StringUtils.isNotBlank(config.getCcAddresses())) {
message.setRecipients(Message.RecipientType.CC, InternetAddress.parse(config.getCcAddresses()));
}
if (StringUtils.isNotBlank(config.getBccAddresses())) {
message.setRecipients(Message.RecipientType.BCC, InternetAddress.parse(config.getBccAddresses()));
}
message.setSubject(emailSubject);
BodyPart messageBodyPart = new MimeBodyPart();
messageBodyPart.setContent(emailBody, "text/html;charset=UTF-8");
Multipart multipart = new MimeMultipart();
multipart.addBodyPart(messageBodyPart);
if (emailAttachment != null && emailAttachment.exists()) {
messageBodyPart = new MimeBodyPart();
DataSource source = new FileDataSource(emailAttachment);
messageBodyPart.setDataHandler(new DataHandler(source));
messageBodyPart.setFileName(emailAttachment.getName());
multipart.addBodyPart(messageBodyPart);
}
message.setContent(multipart);
Transport.send(message);
LOGGER.info("Sent message successfully....");
} catch (Exception e) {
LOGGER.log(Level.SEVERE, "Exception EmailService sendEmailWithAttachment()", e);
throw new EmailServiceException("Error sending email", e);
}
} else {
LOGGER.warning("Email subject or body is empty. Email not sent.");
}
}
@Override
public String getEmailBody() {
return emailBody;
}
@Override
public void setEmailBody(String emailBody) {
this.emailBody = emailBody;
}
@Override
public String getEmailSubject() {
return emailSubject;
}
@Override
public void setEmailSubject(String emailSubject) {
this.emailSubject = emailSubject;
}
@Override
public File getEmailAttachment() {
return emailAttachment;
}
@Override
public void setEmailAttachment(File emailAttachment) {
this.emailAttachment = emailAttachment;
}
@Override
public String getEmailDownloadableLink() {
return emailDownloadableLink;
}
@Override
public void setEmailDownloadableLink(String emailDownloadableLink) {
this.emailDownloadableLink = emailDownloadableLink;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy