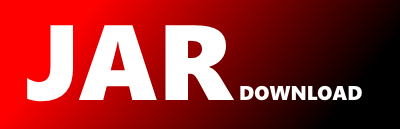
com.ch.model.ExecutionReport Maven / Gradle / Ivy
package com.ch.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Collections;
import java.util.Date;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import com.ch.status.ExecutionStatus;
import com.ch.util.DateTimeUtils;
public class ExecutionReport extends BaseEntity implements Serializable, MetaDataInfo, AdditionalInfo {
/**
*
*/
private static final long serialVersionUID = 4920957033021684153L;
private String executionStartTime;
private String executionEndTime;
private String totalExecutionTime;
private ExecutionStatus status;
private String project;
private String site;
private String subscriptionKey;
private String user;
private String email;
private String scheduledDateTime;
private String triggeredDateTime;
private int expectedTestCounts;
private EnvironmentInfo environmentInfo;
private String timezone = DateTimeUtils.getSystemTimeZone();
transient private Date startDateTime;
transient private Date endDateTime;
private final Map metaDataMap = Collections.synchronizedMap(new ConcurrentHashMap<>());
private final Map additionalInfoMap = Collections.synchronizedMap(new ConcurrentHashMap<>());
public ExecutionReport(String executionId) {
super(executionId);
status = ExecutionStatus.STARTED;
setStartDateTime( new Date());
}
public String getExecutionStartTime() {
return executionStartTime;
}
public String getExecutionEndTime() {
return executionEndTime;
}
public String getTotalExecutionTime() {
return totalExecutionTime;
}
public ExecutionStatus getStatus() {
return status;
}
public void setStatus(ExecutionStatus status) {
this.status = status;
if (status != null && status.equals(ExecutionStatus.COMPLETED)) {
setEndDateTime(new Date());
}
}
/**
* @return the project
*/
public String getProject() {
return project;
}
/**
* @param project the project to set
*/
public void setProject(String project) {
this.project = project;
}
/**
* @return the user
*/
public String getUser() {
return user;
}
/**
* @return the email
*/
public String getEmail() {
return email;
}
/**
* @return the scheduledDateTime
*/
public String getScheduledDateTime() {
return scheduledDateTime;
}
/**
* @param scheduledDateTime the scheduledDateTime to set
*/
public void setScheduledDateTime(String scheduledDateTime) {
this.scheduledDateTime = scheduledDateTime;
}
/**
* @return the triggeredDateTime
*/
public String getTriggeredDateTime() {
return triggeredDateTime;
}
/**
* @param triggeredDateTime the triggeredDateTime to set
*/
public void setTriggeredDateTime(String triggeredDateTime) {
this.triggeredDateTime = triggeredDateTime;
}
/**
* @return the startDateTime
*/
@Transient
public Date getStartDateTime() {
return startDateTime;
}
/**
* @param startDateTime the startDateTime to set
*/
public void setStartDateTime(Date startDateTime) {
this.startDateTime = startDateTime;
if (this.startDateTime == null) {
throw new NullPointerException("StartDateTime is mandatoy");
}
this.executionStartTime = DateTimeUtils.convertDateToUTC(this.startDateTime);
}
/**
* @return the endDateTime
*/
@Transient
public Date getEndDateTime() {
return endDateTime;
}
/**
* @param endDateTime the endDateTime to set
*/
public void setEndDateTime(Date endDateTime) {
this.endDateTime = endDateTime;
if (this.endDateTime == null) {
throw new NullPointerException("EndDateTime is mandatoy");
}
status = ExecutionStatus.COMPLETED;
this.executionEndTime = DateTimeUtils.convertDateToUTC(this.endDateTime);
setTotalExecutionTime();
}
/**
* @return the expectedTestCounts
*/
public int getExpectedTestCounts() {
return expectedTestCounts;
}
/**
* @param expectedTestCounts the expectedTestCounts to set
*/
public void setExpectedTestCounts(int expectedTestCounts) {
this.expectedTestCounts = expectedTestCounts;
}
@Override
public Map getMetaData() {
return metaDataMap;
}
@Override
public void setMetaData(Map metaData) {
if (metaData != null && !metaData.isEmpty()) {
metaDataMap.putAll(metaData);
}
}
@Override
public Map getAdditionalInfo() {
return additionalInfoMap;
}
@Override
public void setAdditionalInfo(Map additionalInfo) {
if (additionalInfo != null && !additionalInfo.isEmpty()) {
additionalInfoMap.putAll(additionalInfo);
}
}
private void setTotalExecutionTime() {
if (this.startDateTime != null && this.endDateTime != null) {
this.totalExecutionTime = DateTimeUtils.calculateEndTime(startDateTime, endDateTime);
} else {
throw new NullPointerException("Property startDateTime or endDateTime is not set");
}
}
public String getSubscriptionKey() {
return subscriptionKey;
}
public void setSubscriptionKey(String subscriptionKey) {
this.subscriptionKey = subscriptionKey;
}
/**
* @return the timezone
*/
public String getTimezone() {
return timezone;
}
public String getSite() {
return site;
}
public void setSite(String site) {
this.site = site;
}
public EnvironmentInfo getEnvironmentInfo() {
return environmentInfo;
}
public void setEnvironmentInfo(EnvironmentInfo environmentInfo) {
this.environmentInfo = environmentInfo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy