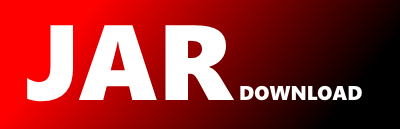
com.ch.model.StepInfo Maven / Gradle / Ivy
package com.ch.model;
import java.io.Serializable;
import java.util.Date;
import com.ch.status.TestStatus;
import com.ch.util.DateTimeUtils;
public class StepInfo implements Serializable {
private static final long serialVersionUID = -3690764012141784427L;
private String stepStartDateTime;
private String stepEndDateTime;
private TestStatus stepStatus;
private String stepName;
private String stepDescription;
private Integer stepSeq = -1;
private MediaInfo stepMedia;
private ExceptionInfo stepException;
transient private Date startDateTime;
transient private Date endDateTime;
public StepInfo(String stepName) {
setStepName(stepName);
setStartDateTime(new Date());
setEndDateTime(new Date());
setStepStatus(TestStatus.INFO);
}
public StepInfo (String stepName, TestStatus stepStatus) {
setStepName(stepName);
setStartDateTime(new Date());
setEndDateTime(new Date());
setStepStatus(stepStatus);
}
public StepInfo (String stepName, TestStatus stepStatus, Throwable t) {
setStepName(stepName);
setStartDateTime(new Date());
setEndDateTime(new Date());
setStepStatus(stepStatus);
ExceptionInfo exceptionInfo = new ExceptionInfo(t);
this.setStepException(exceptionInfo);
}
/**
* @return the stepStartDateTime
*/
public String getStepStartDateTime() {
return stepStartDateTime;
}
/**
* @return the stepEndDateTime
*/
public String getStepEndDateTime() {
return stepEndDateTime;
}
/**
* @return the stepStatus
*/
public TestStatus getStepStatus() {
return stepStatus;
}
/**
* @param stepStatus the stepStatus to set
*/
public void setStepStatus(TestStatus stepStatus) {
this.stepStatus = stepStatus;
}
/**
* @return the stepName
*/
public String getStepName() {
return stepName;
}
/**
* @param stepName the stepName to set
*/
public void setStepName(String stepName) {
this.stepName = stepName;
}
/**
* @return the stepDescription
*/
public String getStepDescription() {
return stepDescription;
}
/**
* @param stepDescription the stepDescription to set
*/
public void setStepDescription(String stepDescription) {
this.stepDescription = stepDescription;
}
/**
* @return the stepSeq
*/
public Integer getStepSeq() {
return stepSeq;
}
/**
* @return the stepMedia
*/
public MediaInfo getStepMedia() {
return stepMedia;
}
/**
* @param stepMedia the stepMedia to set
*/
public void setStepMedia(MediaInfo stepMedia) {
this.stepMedia = stepMedia;
}
/**
* @return the stepException
*/
public ExceptionInfo getStepException() {
return stepException;
}
/**
* @param stepException the stepException to set
*/
public void setStepException(ExceptionInfo stepException) {
this.stepException = stepException;
}
/**
* @param startDateTime the startDateTime to set
*/
public void setStartDateTime(Date startDateTime) {
this.startDateTime = startDateTime;
if (this.startDateTime == null) {
throw new NullPointerException("StartDateTime cannot be null");
}
this.stepStartDateTime = DateTimeUtils.convertDateToUTC(this.startDateTime);
}
/**
* @param endDateTime the endDateTime to set
*/
public void setEndDateTime(Date endDateTime) {
this.endDateTime = endDateTime;
if (this.endDateTime == null) {
throw new NullPointerException("EndDateTime can be null");
}
this.stepEndDateTime = DateTimeUtils.convertDateToUTC(this.endDateTime);
}
public void setStepSeq(int seqNumber) {
this.stepSeq = seqNumber;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy