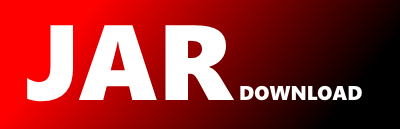
com.ch.model.TestReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ch-model Show documentation
Show all versions of ch-model Show documentation
Defines model classes used for report data.
package com.ch.model;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import com.ch.exception.ValidationException;
import com.ch.status.TestStatus;
import com.ch.util.DateTimeUtils;
public class TestReport extends BaseEntity implements Serializable, MetaDataInfo, AdditionalInfo {
private static final long serialVersionUID = -1738627633194068929L;
public TestReport(String executionId) {
super(executionId);
setStartDateTime(new Date());
}
public TestReport(String testName, TestStatus status, String executionId) {
super(executionId);
setTestName(testName);
setStartDateTime(new Date());
setStatus(status);
}
public TestReport(String testCaseName, String executionId) {
super(executionId);
this.testName = testCaseName;
setStartDateTime(new Date());
}
private String testName;
private String testId;
private String testDescription;
private String[] testGroups;
private String moduleName;
private String suiteName;
private String priority;
private String executionTime;
private String startTime;
private String endTime;
private MediaInfo attachment; // Base64 encode image
private TestStatus status;
private ExceptionInfo exceptionInfo;
private EnvironmentInfo environmentInfo;
private String userStoryId;
private int noOfRetries;
private final List steps = Collections.synchronizedList(new ArrayList<>());
private String timezone = DateTimeUtils.getSystemTimeZone();
private String subscriptionKey;
private String user;
transient private Date startDateTime;
transient private Date endDateTime;
private final Map metaDataMap = Collections.synchronizedMap(new ConcurrentHashMap<>());
private final Map additionalInfoMap = Collections.synchronizedMap(new ConcurrentHashMap<>());
private final void addStep(StepInfo step) {
addStep(step, steps);
}
private final void addStep(StepInfo step, List list) {
step.setStepSeq(list.size());
list.add(step);
}
public String[] getTestGroups() {
return testGroups;
}
public void setTestGroups(String[] testGroups) {
this.testGroups = testGroups;
}
public String getModuleName() {
return moduleName;
}
public void setModuleName(String moduleName) {
this.moduleName = moduleName;
}
public String getSuiteName() {
return suiteName;
}
public void setSuiteName(String suiteName) {
this.suiteName = suiteName;
}
public String getPriority() {
return priority;
}
public void setPriority(String priority) {
this.priority = priority;
}
public String getExecutionTime() {
return executionTime;
}
public String getStartTime() {
return startTime;
}
public String getEndTime() {
return endTime;
}
public MediaInfo getAttachment() {
return attachment;
}
public void setAttachment(MediaInfo attachment) {
this.attachment = attachment;
}
public ExceptionInfo getExceptionInfo() {
return exceptionInfo;
}
public void setExceptionInfo(ExceptionInfo exceptionInfo) {
this.exceptionInfo = exceptionInfo;
}
public EnvironmentInfo getEnvironmentInfo() {
return environmentInfo;
}
public void setEnvironmentInfo(EnvironmentInfo environmentInfo) {
this.environmentInfo = environmentInfo;
}
public List getSteps() {
return steps;
}
public String getTestName() {
return testName;
}
public void setTestName(String testName) {
this.testName = testName;
}
public String getTestId() {
return testId;
}
public void setTestId(String testId) {
this.testId = testId;
}
public String getTestDescription() {
return testDescription;
}
public void setTestDescription(String testDescription) {
this.testDescription = testDescription;
}
public TestStatus getStatus() {
return status;
}
public String getUserStoryId() {
return userStoryId;
}
public void setUserStoryId(String userStoryId) {
this.userStoryId = userStoryId;
}
public int getNoOfRetries() {
return noOfRetries;
}
public void setNoOfRetries(int noOfRetries) {
this.noOfRetries = noOfRetries;
}
public String getTimezone() {
return timezone;
}
public Date getStartDateTime() {
return startDateTime;
}
public String getSubscriptionKey() {
return subscriptionKey;
}
public void setSubscriptionKey(String subscriptionKey) {
this.subscriptionKey = subscriptionKey;
}
public void setStartDateTime(Date startDateTime) {
this.startDateTime = startDateTime;
if (this.startDateTime == null) {
throw new NullPointerException("StartDateTime is mandatoy");
}
this.startTime = DateTimeUtils.convertDateToUTC(this.startDateTime);
}
public Date getEndDateTime() {
return endDateTime;
}
public void setEndDateTime(Date endDateTime) {
this.endDateTime = endDateTime;
if (this.endDateTime == null) {
throw new NullPointerException("EndDateTime is mandatoy");
}
this.endTime = DateTimeUtils.convertDateToUTC(this.endDateTime);
if (this.startDateTime != null && this.endDateTime != null) {
this.executionTime = DateTimeUtils.calculateEndTime(startDateTime, endDateTime);
} else {
throw new NullPointerException("Property startDateTime or endDateTime is not set");
}
}
public StepInfo createStepInfo(String stepName) {
StepInfo stepInfo = new StepInfo(stepName);
addStep(stepInfo);
return stepInfo;
}
public StepInfo createStepInfo(String stepName, TestStatus stepStatus) {
StepInfo stepInfo = new StepInfo(stepName, stepStatus);
addStep(stepInfo);
return stepInfo;
}
public StepInfo createStepInfo(String stepName, TestStatus stepStatus, Throwable t) {
StepInfo stepInfo = new StepInfo(stepName, stepStatus, t);
addStep(stepInfo);
return stepInfo;
}
@Override
public Map getMetaData() {
return metaDataMap;
}
@Override
public void setMetaData(Map metaData) {
if (metaData != null && !metaData.isEmpty()) {
metaDataMap.putAll(metaData);
}
}
@Override
public Map getAdditionalInfo() {
return additionalInfoMap;
}
@Override
public void setAdditionalInfo(Map additionalInfo) {
if (additionalInfo != null && !additionalInfo.isEmpty()) {
additionalInfoMap.putAll(additionalInfo);
}
}
public void setStatus(TestStatus status) {
this.status = status;
setEndDateTime(new Date());
}
public void setStatus(TestStatus status, Throwable t) {
this.status = status;
this.exceptionInfo = new ExceptionInfo(t);
}
public void setStatus(TestStatus status, String imageBase64String, Throwable t) throws ValidationException {
this.status = status;
this.exceptionInfo = new ExceptionInfo(t);
this.attachment = new MediaInfo(imageBase64String);
}
public String getUser() {
return user;
}
public void setUser(String user) {
this.user = user;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy