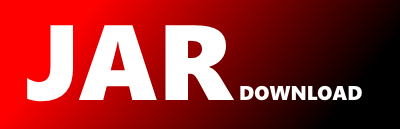
com.ch.dao.ExecutionDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ch-mongo Show documentation
Show all versions of ch-mongo Show documentation
Persistence services for mongo collections.
The newest version!
package com.ch.dao;
import java.text.MessageFormat;
import java.util.Date;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.bson.Document;
import com.ch.config.ConfigProperties;
import com.ch.constants.Constant;
import com.ch.exception.DAOException;
import com.ch.model.ExecutionReport;
import com.ch.mongo.TemplateDao;
import com.fasterxml.jackson.databind.MapperFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.mongodb.client.FindIterable;
import com.mongodb.client.MongoCursor;
public class ExecutionDao extends TemplateDao {
private static final Logger LOGGER = Logger.getLogger(ExecutionDao.class.getName());
String collectioName = null;
public ExecutionDao(ConfigProperties config) throws Exception {
super(config);
collectioName = Constant.EXECUTION_REPORT_COLLECTION_NAME;
}
/** If an update operation with upsert: true results in an insert of a document,
* then $setOnInsert assigns the specified values to the fields in the document.
* If the update operation does not result in an insert, $setOnInsert does nothing.
*
*/
@Override
public void save(ExecutionReport execution) throws DAOException {
try {
Document filter = new Document();
filter.append("executionId", execution.getExecutionId());
filter.append("subscriptionKey", execution.getSubscriptionKey());
LOGGER.finest("filter query -> "+filter.toJson());
FindIterable iterableDocument = mongoClient.getDatabase(databaseName).getCollection(collectioName).find(filter).limit(1);
if (iterableDocument != null) {
MongoCursor cursor = iterableDocument.iterator();
if (cursor.hasNext()) {
Document document = cursor.next();
String pExecutionId = document.getString("executionId");
String status = document.getString("status");
if(status != null && status.equalsIgnoreCase("completed")) {
String msg = MessageFormat.format("executionId {0} already in completed status. Cannot reuse same Id",pExecutionId);
throw new DAOException(msg);
}
LOGGER.finest(" Got executionId from DB "+ pExecutionId);
Document updatedDocument = new Document();
updatedDocument.put("status", execution.getStatus().toString());
updatedDocument.put("updatedAt", new Date());
if(execution.getMetaData() != null && !execution.getMetaData().isEmpty()) {
updatedDocument.put("metaData", execution.getMetaData());
}
if(execution.getAdditionalInfo() != null && !execution.getAdditionalInfo().isEmpty()) {
updatedDocument.put("additionalInfo", execution.getAdditionalInfo());
}
updatedDocument.put("executionEndTime", execution.getExecutionEndTime());
updatedDocument.put("totalExecutionTime", execution.getTotalExecutionTime());
updatedDocument.put("expectedTestCounts", execution.getExpectedTestCounts());
ObjectMapper mapper = new ObjectMapper();
mapper.enable(SerializationFeature.INDENT_OUTPUT);
mapper.enable(MapperFeature.PROPAGATE_TRANSIENT_MARKER);
String environmentInfo = mapper.writeValueAsString(execution.getEnvironmentInfo());
String additionalInfo = mapper.writeValueAsString(execution.getAdditionalInfo());
String metaInfo = mapper.writeValueAsString(execution.getMetaData());
updatedDocument.put("environmentInfo", Document.parse(environmentInfo));
updatedDocument.put("additionalInfo", Document.parse(additionalInfo));
updatedDocument.put("metaData", Document.parse(metaInfo));
//Need to wrap document on another document with "$set" to avoid "invalid BSON field"
mongoClient.getDatabase(databaseName).getCollection(collectioName).updateOne(filter,new Document("$set", updatedDocument));
} else {
//Insert below fields if record is being created
ObjectMapper mapper = new ObjectMapper();
mapper.enable(SerializationFeature.INDENT_OUTPUT);
//mapper.setSerializationInclusion(Include.NON_NULL);
mapper.enable(MapperFeature.PROPAGATE_TRANSIENT_MARKER);
String executionReportJson = mapper.writeValueAsString(execution);
LOGGER.finest("executionReportJson -->" + executionReportJson);
Document newDocument = Document.parse(executionReportJson);
newDocument.put("updatedAt", new Date());
newDocument.put("createdAt", new Date());
mongoClient.getDatabase(databaseName).getCollection(collectioName).insertOne(newDocument);
}
}
LOGGER.info("Execution Report data saved successfully.");
} catch (Exception ex) {
LOGGER.log(Level.SEVERE, ex.getMessage(), ex);
throw new DAOException("Exception while saving the Execution into collection", ex);
}
}
@Override
public void update(ExecutionReport executionDetails, String[] params) throws DAOException {
throw new DAOException("Method not implemented");
}
@Override
public void delete(ExecutionReport t) throws DAOException {
throw new DAOException("Method not implemented");
}
@Override
public void save(List t) throws DAOException {
ExecutionReport executionReport = t.get(0);
save(executionReport);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy