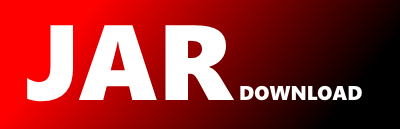
com.ch.dao.TestCaseDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ch-mongo Show documentation
Show all versions of ch-mongo Show documentation
Persistence services for mongo collections.
The newest version!
package com.ch.dao;
import java.util.Date;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.bson.Document;
import com.ch.config.ConfigProperties;
import com.ch.constants.Constant;
import com.ch.exception.DAOException;
import com.ch.model.StepInfo;
import com.ch.model.TestReport;
import com.ch.model.mixin.StepInfoMixIn;
import com.ch.model.mixin.TestReportMixIn;
import com.ch.mongo.TemplateDao;
import com.fasterxml.jackson.databind.MapperFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
public class TestCaseDao extends TemplateDao {
private static final Logger LOGGER = Logger.getLogger(TestCaseDao.class.getName());
String collectioName = null;
public TestCaseDao(ConfigProperties config) throws Exception {
super(config);
collectioName = Constant.TEST_REPORT_COLLECTION_NAME;
}
@Override
public void save(TestReport t) throws DAOException {
try {
ObjectMapper mapper = new ObjectMapper();
mapper.enable(SerializationFeature.INDENT_OUTPUT);
//mapper.setSerializationInclusion(Include.NON_NULL);
mapper.enable(MapperFeature.PROPAGATE_TRANSIENT_MARKER);
//default Mixin is enabled. Mixin allows to compact JSON by removing image string ( base64 encode) from payload
boolean disableMixin = false;
try {
String disableMixinFlagStr = configProperties.getProperty("DISABLE_MIXIN");
if (disableMixinFlagStr != null && disableMixinFlagStr.trim().equalsIgnoreCase("true")) {
disableMixin = true;
}
} catch(Exception e) {
disableMixin = false;
}
if(!disableMixin) {
//removed base64 image strings from serializing into JSON object
mapper.addMixIn(TestReport.class, TestReportMixIn.class);
mapper.addMixIn(StepInfo.class, StepInfoMixIn.class);
}
String testCaseJson = mapper.writeValueAsString(t);
LOGGER.finest("Test report document to persist " + testCaseJson);
Document doc = Document.parse(testCaseJson);
doc.put("updatedAt", new Date());
doc.put("createdAt", new Date());
mongoClient.getDatabase(databaseName).getCollection(collectioName).insertOne(doc);
LOGGER.finest("Test Report data saved successfully.");
} catch (Exception ex) {
LOGGER.log(Level.SEVERE, ex.getMessage(), ex);
throw new DAOException("Exception while saving the TestReport into collection", ex);
}
}
@Override
public void update(TestReport t, String[] params) throws DAOException {
throw new DAOException("Method not implemented");
}
@Override
public void delete(TestReport t) throws DAOException {
throw new DAOException("Method not implemented");
}
@Override
public void save(List t) throws DAOException {
for (TestReport report : t) {
save(report);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy